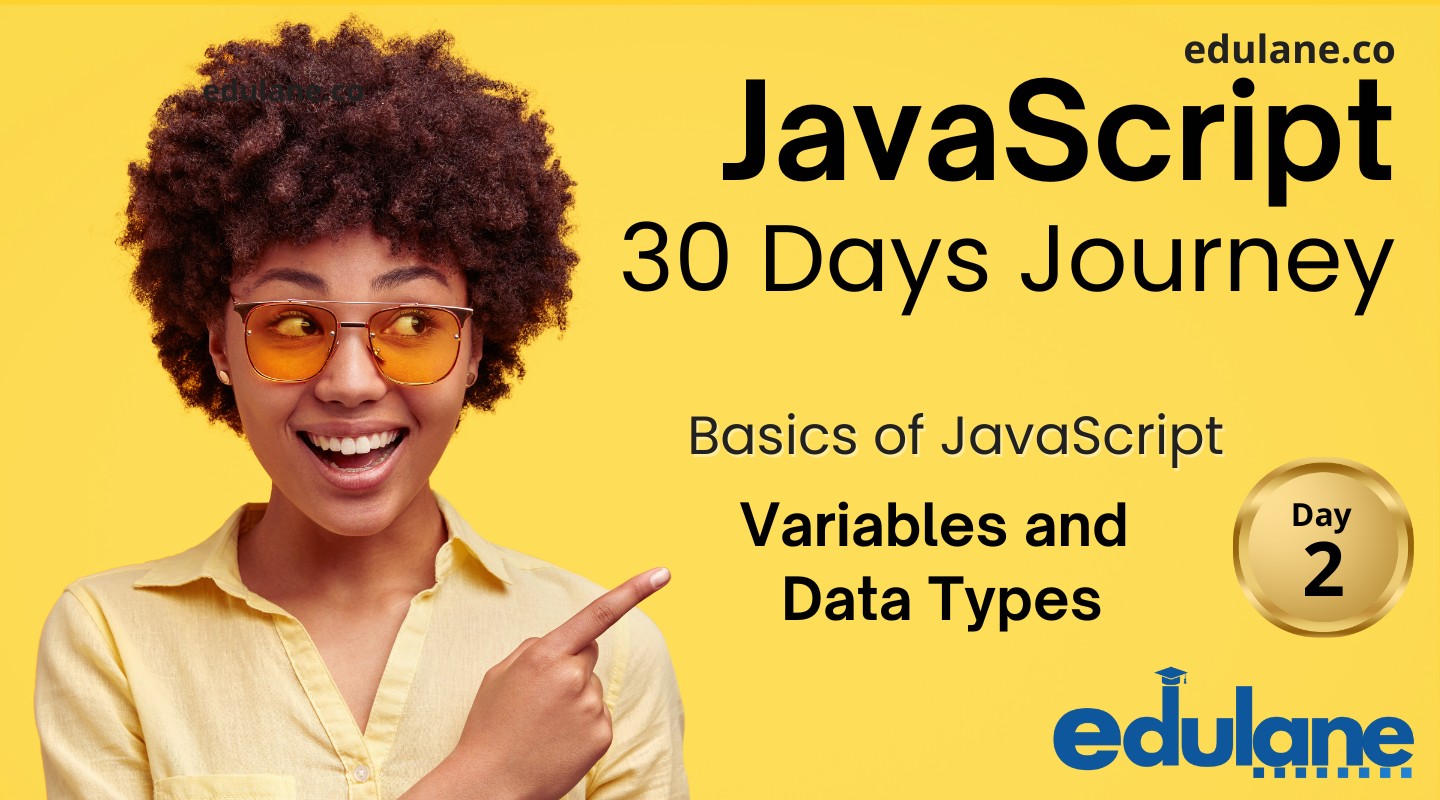
JavaScript Variables and Data Types : A Detailed Guide
JavaScript Basics of Variables
In JavaScript, a variable is a named storage location that holds data values. These values can vary and can be changed during the execution of a program. Variables are fundamental to programming because they allow developers to store and manipulate data dynamically rather than hard-coding values into the program.
Variables are used to store data that can be used and manipulated throughout your code. In JavaScript, you can declare variables using var
, let
, and const
.
var
is function-scoped and can be re-declared and updated.let
is block-scoped and can be updated but not re-declared within the same scope.const
is block-scoped and cannot be updated or re-declared; it is used for variables that should not change.
- Declaration: Variables in JavaScript are declared using
var
,let
, orconst
keywords.var
: Historically used to declare variables. Variables declared withvar
are function-scoped or globally scoped if declared outside a function.let
: Introduced in ES6 (ECMAScript 2015) and is block-scoped (limited to the block in which it is defined, like loops or conditionals).const
: Also introduced in ES6,const
declares variables that are block-scoped likelet
, but its value cannot be reassigned once set (though the value itself can be mutable, such as arrays or objects).
- Initialization: Variables can be declared and assigned a value simultaneously.
var age = 30;
let firstName = "John";
const PI = 3.14;
- Dynamic Typing: JavaScript is dynamically typed, meaning variables can hold values of any data type without any explicit type declaration.
let message = "Hello"; // message is a string
message = 123; // now message is a number
- Scope: The scope of a variable defines where in your code that variable is accessible.
- Variables declared with
var
have function scope or global scope. - Variables declared with
let
andconst
have block scope.
- Variables declared with
- Hoisting: In JavaScript, variable declarations (not initialization) are hoisted to the top of their containing scope.
console.log(x); // undefined
var x = 5;
This behavior can lead to unexpected results if not understood properly.
- Naming conventions: Variables in JavaScript must follow certain naming rules:
- Names can contain letters, digits, underscores, and dollar signs.
- Names must begin with a letter,
$
, or_
. - Names are case-sensitive (
age
,Age
, andAGE
are different variables).

JavaScript Variables Examples
- Example 1: Using
var
// 1. Basic Declaration and Initialization:
var greeting = "Hello, World!";
console.log(greeting); // Output: Hello, World!
// Explanation: A variable greeting is declared and initialized with the value "Hello, World!".
// 2. Reassignment:
var age = 25;
console.log(age); // Output: 25
age = 30;
console.log(age); // Output: 30
// Explanation: The variable age is reassigned from 25 to 30.
// 3. Variable Hoisting:
console.log(hoistedVar); // Output: undefined
var hoistedVar = "I am hoisted!";
console.log(hoistedVar); // Output: I am hoisted!
// Explanation: Variable declarations with var are hoisted to the top of their scope, but the initialization stays in place.
// 4. Function Scope:
function scopeTest() {
var localVar = "I am local";
console.log(localVar); // Output: I am local
}
scopeTest();
// console.log(localVar); // Error: localVar is not defined
// Explanation: var is function-scoped, so localVar is not accessible outside the function.
// 5. Global Scope:
var globalVar = "I am global";
function testGlobalScope() {
console.log(globalVar); // Output: I am global
}
testGlobalScope();
// Explanation: var declared outside any function is globally scoped.
// 6. Redeclaration:
var message = "Hello";
var message = "World";
console.log(message); // Output: World
// Explanation: Variables declared with var can be redeclared without any issues.
// 7. Inside Loops:
for (var i = 0; i < 3; i++) {
console.log(i);
}
console.log(i); // Output: 3
// Explanation: var is not block-scoped, so i is accessible outside the loop.
// 8. Inside Conditional Blocks:
if (true) {
var conditionVar = "I am inside an if block";
}
console.log(conditionVar); // Output: I am inside an if block
// Explanation: var is not block-scoped, so conditionVar i10s accessible outside the if block.
// 9. Variable Hoisting in Functions:
function hoistExample() {
console.log(hoisted); // Output: undefined
var hoisted = "This is hoisted";
console.log(hoisted); // Output: This is hoisted
}
hoistExample();
// Explanation: Within a function, var declarations are hoisted to the top.
// 10. Variable Shadowing:
var outer = "I am outside";
function shadowingExample() {
var outer = "I am inside";
console.log(outer); // Output: I am inside
}
shadowingExample();
console.log(outer); // Output: I am outside
// Explanation: The inner var shadows the outer var within the function.
// 11. Using var in Nested Functions:
function outerFunction() {
var outerVar = "I am outside";
function innerFunction() {
var innerVar = "I am inside";
console.log(outerVar); // Output: I am outside
console.log(innerVar); // Output: I am inside
}
innerFunction();
// console.log(innerVar); // Error: innerVar is not defined
}
outerFunction();
// Explanation: Inner function can access outer function's var variables, but not vice versa.
// 12. Variable Scope in Closures:
function createCounter() {
var count = 0;
return function() {
count++;
return count;
};
}
var counter = createCounter();
console.log(counter()); // Output: 1
console.log(counter()); // Output: 2
// Explanation: The inner function retains access to the outer function's var variable due to closure.
// 13. Variable Declaration Without Initialization:
var uninitialized;
console.log(uninitialized); // Output: undefined
// Explanation: Declaring a var variable without initialization sets it to undefined.
// 14. Accessing var Before Declaration:
console.log(preDeclared); // Output: undefined
var preDeclared = "I am pre-declared";
// Explanation: Accessing var before its declaration results in undefined due to hoisting.
// 15. Re-declaration in Different Scopes:
var scopeVar = "global scope";
function testScopes() {
var scopeVar = "local scope";
console.log(scopeVar); // Output: local scope
}
testScopes();
console.log(scopeVar); // Output: global scope
// Explanation: Re-declaring var in different scopes creates separate instances for each scope.
- Example 2: Using
let
// 1. Basic Declaration and Initialization:
let greeting = "Hello, World!";
console.log(greeting); // Output: Hello, World!
// Explanation: let greeting declares a variable named greeting and initializes it with the string "Hello, World!". The value is then printed to the console.
// 2. Block Scope:
if (true) {
let message = "This is block-scoped";
console.log(message); // Output: This is block-scoped
}
// console.log(message); // Error: message is not defined
// Explanation: The message variable is declared inside an if block, making it accessible only within that block. Trying to access message outside the block results in an error.
// 3. Reassignment:
let age = 30;
console.log(age); // Output: 30
age = 31;
console.log(age); // Output: 31
// Explanation: let age declares a variable and initializes it with 30. The value of age is then changed to 31, showing that let allows reassignment.
// 4. No Redeclaration:
let firstName = "Alice";
// let name = "Bob"; // Error: Identifier 'name' has already been declared
name = "Bob"; // Correct way to reassign
console.log(name); // Output: Bob
// Explanation: Once a variable is declared with let, it cannot be redeclared in the same scope. Reassignment is allowed, but redeclaration causes an error.
// 5. For Loop Scope:
for (let i = 0; i < 5; i++) {
console.log(i); // Output: 0, 1, 2, 3, 4
}
// console.log(i); // Error: i is not defined
// Explanation: let i inside the for loop is block-scoped, meaning i is only accessible within the loop. Accessing i outside the loop causes an error.
// 6. Nested Block Scope:
let outer = "I am outside";
{
let inner = "I am inside";
console.log(inner); // Output: I am inside
}
console.log(outer); // Output: I am outside
// console.log(inner); // Error: inner is not defined
/*
Explanation:
outer is declared outside any block and is accessible everywhere in the outer scope.
inner is declared inside a block and is only accessible within that block.
*/
// 7. Switch Case Scope:
let fruit = "apple";
switch (fruit) {
case "apple":
let color = "red";
console.log(color); // Output: red
break;
case "banana":
let color = "yellow";
console.log(color); // Output: yellow
break;
}
// console.log(color); // Error: color is not defined
// Explanation: The let color declaration is scoped to each case block within the switch statement, preventing access outside those blocks.
// 8. Function Parameters:
function greet(name) {
let message = `Hello, ${name}!`;
console.log(message); // Output: Hello, [name]!
}
greet("Alice"); // Output: Hello, Alice!
// Explanation: The name parameter is passed to the function and used within the function to create a message variable, which is then printed.
// 9. Temporal Dead Zone:
// console.log(tempVar); // Error: Cannot access 'tempVar' before initialization
let tempVar = "I exist now";
console.log(tempVar); // Output: I exist now
// Explanation: The let variable tempVar cannot be accessed before its declaration due to the temporal dead zone, which is the time between entering the block and the actual declaration.
// 10. Block Scope with Conditionals:
let x = 10;
if (x > 5) {
let y = x * 2;
console.log(y); // Output: 20
}
// console.log(y); // Error: y is not defined
// Explanation: The variable y is declared inside an if block and is only accessible within that block. Accessing y outside the block causes an error.
// 11. Using let in Functions:
function calculateArea(radius) {
let area = Math.PI * radius * radius;
return area;
}
console.log(calculateArea(5)); // Output: 78.53981633974483
// Explanation: The variable area is declared inside the function calculateArea and is used to calculate and return the area of a circle.
// 12. Nested Functions:
function outerFunction() {
let outerVar = "I am outside";
function innerFunction() {
let innerVar = "I am inside";
console.log(outerVar); // Output: I am outside
}
innerFunction();
// console.log(innerVar); // Error: innerVar is not defined
}
outerFunction();
/*
Explanation:
outerVar is declared in the outer function and is accessible inside the inner function.
innerVar is declared in the inner function and is only accessible within it.
*/
// 13. Block Scope in Loops:
let results = [];
for (let i = 0; i < 5; i++) {
let result = i * 2;
results.push(result);
}
console.log(results); // Output: [0, 2, 4, 6, 8]
// console.log(result); // Error: result is not defined
// Explanation: The variable result is declared inside the for loop and is only accessible within each iteration of the loop.
// 14. Shadowing Variables:
let name = "Alice";
{
let name = "Bob";
console.log(name); // Output: Bob
}
console.log(name); // Output: Alice
// Explanation: The name variable declared inside the block shadows the name variable outside the block, meaning the inner name is used within the block, and the outer name is used outside the block.
// 15. Closure with let:
function createCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const counter = createCounter();
console.log(counter()); // Output: 1
console.log(counter()); // Output: 2
// Explanation: The variable count is declared in the outer function createCounter and is accessible in the returned inner function, creating a closure that maintains the state of count across multiple calls.
- Example 3: Using
const
// 1. Basic Declaration and Initialization:
const greeting = "Hello, World!";
console.log(greeting); // Output: Hello, World!
// Explanation: const greeting declares a constant variable named greeting and initializes it with the string "Hello, World!". Constants are conventionally named in all uppercase with underscores separating words for readability, but for single words, camelCase is also acceptable.
// 2. Constant Primitive Value:
const PI = 3.14159;
console.log(PI); // Output: 3.14159
// Explanation: const PI declares a constant variable named PI and initializes it with the value 3.14159, representing the mathematical constant Pi. Constants that represent mathematical or logical values are often named in all uppercase.
// 3. Reassignment Error:
const age = 25;
// age = 30; // Error: Assignment to constant variable.
console.log(age); // Output: 25
// Explanation: const age declares a constant variable named age. Attempting to reassign a value to age results in an error because constants cannot be reassigned once initialized.
// 4. Constant Object:
const person = { name: "Alice", age: 30 };
console.log(person); // Output: { name: "Alice", age: 30 }
// Explanation: const person declares a constant variable named person and initializes it with an object containing properties name and age. Although the object itself is constant, its properties can be modified.
// 5. Modifying Object Properties:
const car = { brand: "Toyota", model: "Corolla" };
car.model = "Camry";
console.log(car); // Output: { brand: "Toyota", model: "Camry" }
// Explanation: The properties of const car can be modified, but the reference to the object cannot be changed after initialization.
// 6. Constant Array:
const colors = ["red", "green", "blue"];
console.log(colors); // Output: ["red", "green", "blue"]
// Explanation: const colors declares a constant variable named colors and initializes it with an array of strings. While the array itself is constant, its elements can be modified.
// 7. Modifying Array Elements:
const fruits = ["apple", "banana"];
fruits.push("orange");
console.log(fruits); // Output: ["apple", "banana", "orange"]
// Explanation: Elements can be added to const fruits, demonstrating that the array can be modified even though the reference to the array cannot be reassigned.
// 8. Block Scope:
if (true) {
const message = "This is block-scoped";
console.log(message); // Output: This is block-scoped
}
// console.log(message); // Error: message is not defined
// Explanation: The message variable is block-scoped within the if block, meaning it is only accessible within that block and not outside of it.
// 9. Nested Block Scope:
const outer = "I am outside";
{
const inner = "I am inside";
console.log(inner); // Output: I am inside
}
console.log(outer); // Output: I am outside
// console.log(inner); // Error: inner is not defined
/*
Explanation:
const outer is accessible outside the inner block because it's declared in the outer scope.
const inner is only accessible within its block due to block scoping.
*/
// 10. Constant with Function:
const greet = function(name) {
return `Hello, ${name}!`;
};
console.log(greet("Alice")); // Output: Hello, Alice!
// Explanation: const greet declares a constant variable that holds a function expression. While the function can be invoked, the reference to greet cannot be reassigned.
// 11. Constant Function Expression:
const square = (x) => x * x;
console.log(square(5)); // Output: 25
// Explanation: const square declares a constant variable that holds an arrow function expression. Arrow functions assigned to constants cannot have their references reassigned.
// 12. Constant Inside Function:
function calculateArea(radius) {
const PI = 3.14159;
return PI * radius * radius;
}
console.log(calculateArea(5)); // Output: 78.53975
// Explanation: const PI is declared inside the calculateArea function and is scoped to that function. It holds the value of Pi and remains constant within the function's execution.
// 13. Closure with const:
function createCounter() {
let count = 0;
return function() {
const increment = 1;
count += increment;
return count;
};
}
const counter = createCounter();
console.log(counter()); // Output: 1
console.log(counter()); // Output: 2
// Explanation: const increment is declared inside the returned function from createCounter. It maintains its constant value across multiple invocations of the counter function due to closure.
// 14. Constant with Destructuring:
const person = { name: "Alice", age: 30 };
const { name, age } = person;
console.log(name); // Output: Alice
console.log(age); // Output: 30
// Explanation: const { name, age } uses object destructuring to assign values from the person object to constants named name and age. Destructured constants cannot be reassigned.
// 15. Naming Convention:
/*
Constants in JavaScript conventionally use all uppercase letters with words
separated by underscores (CONSTANT_NAME) for improved readability
and to distinguish them from regular variables.
This convention helps developers quickly identify constants within code.
*/
/*
These examples illustrate how to use the const keyword in JavaScript for creating immutable bindings,
ensuring that variables declared with const cannot be reassigned once initialized.
*/
Prepare yourself for interview questions by checking the list below:
- What are the differences between
var
,let
, andconst
? - How does variable hoisting work with
var
,let
, andconst
? - What is the temporal dead zone (TDZ) in JavaScript?
- Can you reassign values to variables declared with
let
andconst
? - What will happen if you try to redeclare a variable with
var
,let
, andconst
? - Why might you choose
let
overvar
? - Can you explain block scope and how it relates to
let
andconst
? - How do
var
,let
, andconst
behave inside a function? - What are the scoping rules for
var
in loops? - What happens if you declare a variable with
const
but do not initialize it immediately? - How does
const
affect object and array variables? - Can you mutate the value of an object or array declared with
const
? - What is the best practice for choosing between
var
,let
, andconst
? - What errors are thrown when trying to access
let
andconst
variables before their declaration? - How do
let
andconst
impact the global object in JavaScript? - What are the benefits of using
const
for variables that do not need reassignment? - How does variable shadowing work with
var
,let
, andconst
? - Can you explain the differences in the way
var
,let
, andconst
handle scope in afor
loop? - What are some common pitfalls of using
var
thatlet
andconst
help to avoid? - Give an example of how to use
const
to ensure the immutability of a variable.
Tips & Best Practices
General Guidelines
- Prefer
let
andconst
overvar
:var
is function-scoped and can lead to unexpected behaviors due to hoisting and scope issues.let
andconst
are block-scoped and generally safer to use.
- Use
const
by Default:- Use
const
for variables that won’t be reassigned. This makes your intentions clear and helps prevent accidental reassignments.
- Use
- Use
let
for Mutable Variables:- Use
let
when you know that the variable’s value will need to change.
- Use
Specific Practices
- Avoid Re-declaration:
- Avoid redeclaring variables in the same scope, which can lead to confusion and errors. Using
let
andconst
helps prevent this, as they do not allow redeclaration in the same scope.
- Avoid redeclaring variables in the same scope, which can lead to confusion and errors. Using
- Minimize Variable Scope:
- Declare variables in the closest scope possible to where they are used. This improves readability and reduces potential errors.
- Initialize Variables:
- Always initialize variables when you declare them. This avoids issues with
undefined
values and improves code clarity.
- Always initialize variables when you declare them. This avoids issues with
- Use Meaningful Names:
- Choose clear, descriptive names for your variables. This makes your code more readable and maintainable.
Best Practices in Context
- Handling Objects and Arrays with
const
:- While
const
prevents reassignment, it doesn’t make the value immutable. For objects and arrays, you can still modify their contents. If immutability is required, consider using libraries like Immutable.js or use cloning techniques.
- While
- Avoid Global Variables:
- Minimize the use of global variables to reduce the risk of conflicts and unintended behavior. Encapsulate variables within functions or blocks.
- Hoisting Awareness:
- Understand hoisting behavior with
var
,let
, andconst
.var
declarations are hoisted and initialized withundefined
, whilelet
andconst
are hoisted but not initialized until their declaration is evaluated.
- Understand hoisting behavior with
- Block Scoping in Loops
- Use
let
orconst
within loops to ensure the variable is block-scoped. This prevents issues that can arise withvar
due to its function-scoped nature.
- Use
Code Examples
Using const
for Constants:
const MAX_USERS = 100;
// MAX_USERS = 200; // This will throw an error
Using let
for Mutable Variables:
let count = 0;
count += 1;
Avoiding Variable Re-declaration:
let userName = 'Alice';
// let userName = 'Bob'; // This will throw an error
Proper Scoping in Loops:
for (let i = 0; i < 10; i++) {
console.log(i);
}
// console.log(i); // This will throw an error
By following these best practices/tips, you can write cleaner, more reliable, and more maintainable JavaScript code.