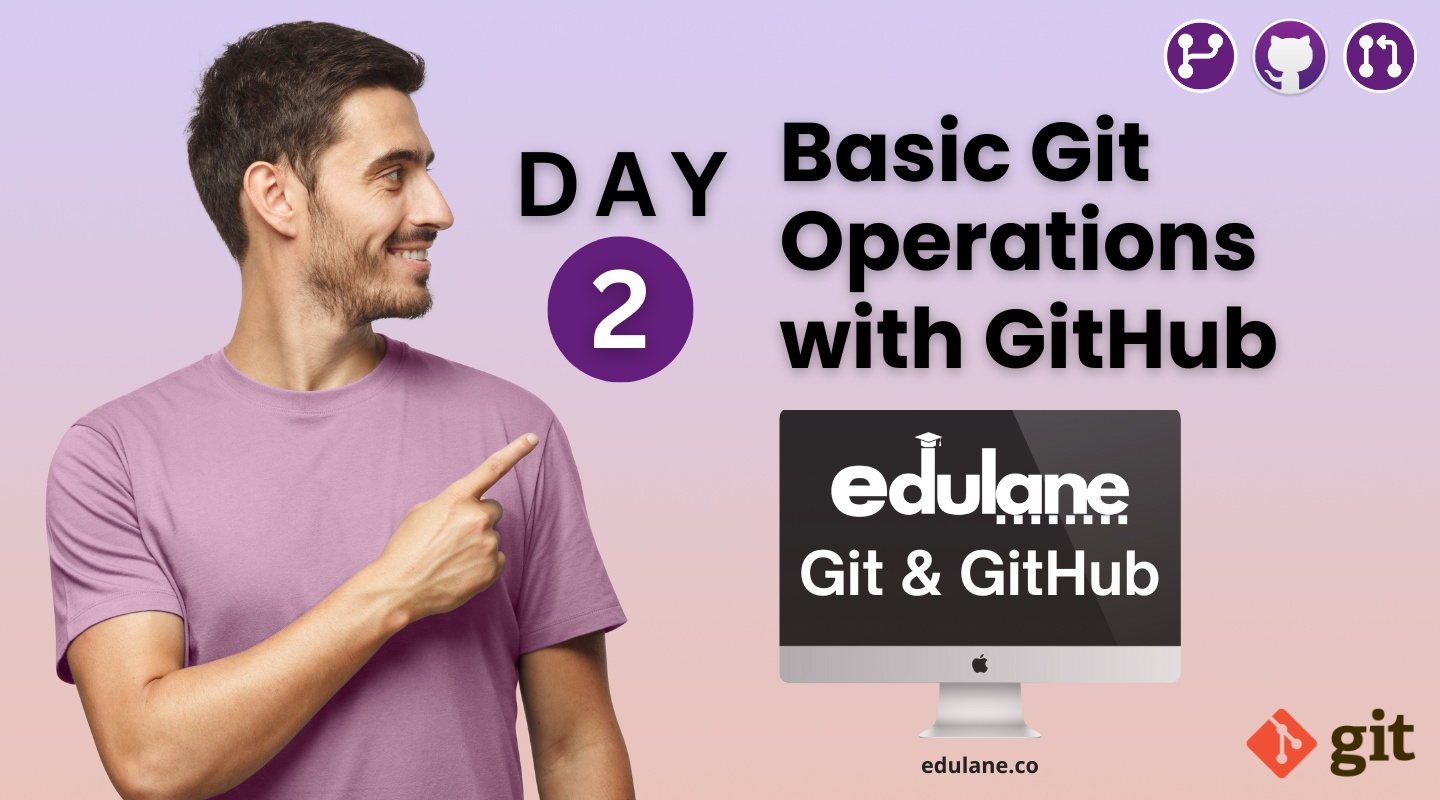
Mastering Basic Git Operations with GitHub CLI: A Comprehensive Beginner’s Guide
Learn how to efficiently manage your GitHub repositories from the command line with this comprehensive guide to Git and GitHub CLI. From installation and configuration to executing basic Git commands like git add
, git commit
, clone
, push
, pull
, and branch management, this tutorial covers everything you need to get started. Understand best practices for using Git and GitHub, streamline your workflow, and enhance your productivity with detailed explanations and real-time examples tailored for beginners. Perfect for developers looking to seamlessly integrate GitHub operations into their command line workflow.
Table of Contents
- Introduction to GitHub CLI
- Installing Git for CLI
- Setting Up GitHub CLI
- Git Configuration
- Basic GitHub CLI Commands
- Pull Request
- Step-by-Step Guide to Making a Pull Request
- Best Practices
- Conclusion
Introduction to GitHub CLI
The GitHub CLI (gh) is a powerful tool that allows you to interact with GitHub from the command line. With GitHub CLI, you can manage repositories, issues, pull requests, and more, all without leaving your terminal.
Installing Git for CLI
Before using GitHub CLI, you need to install Git, which is the version control system that GitHub is built on. Here are the steps to install Git on different operating systems.
Windows
- Go to the Git for Windows download page.
- Click the “Download” button to download the latest installer.
- Run the downloaded installer and follow the instructions. During the installation, you can use the default settings or customize the installation to fit your needs.
- Verify the installation by opening a command prompt and running:
git --version
You should see the installed version of Git.
macOS
- Install Git using Homebrew:
brew install git
- Verify the installation by opening a terminal and running:
git --version
You should see the installed version of Git.
Linux
- Install Git using a package manager. For Debian/Ubuntu-based distributions, run:
sudo apt install git
- For Fedora-based distributions, run:
sudo dnf install git
- Verify the installation by opening a terminal and running
git --version
You should see the installed version of Git.
Setting Up GitHub CLI
Installation
To use GitHub CLI, you need to install it on your system. Follow these steps:
Windows
- Download the installer from the GitHub CLI
- Run the installer and follow the instructions.
macOS
- Install using Homebrew:
brew install gh
Linux
- Install using a package manager (e.g., apt for Debian/Ubuntu):
sudo apt install gh
Authentication
Once installed, you need to authenticate GitHub CLI with your GitHub account:
- Open your terminal.
- Run the following command to authenticate:
gh auth login
- Follow the prompts to complete the authentication process.
Git Configuration
Before you start using Git, you need to configure it with your identity. This information will be used in your commits.
- Set your name:
git config --global user.name "Your Name"
- Set your email:
git config --global user.email "your.email@example.com"
- Verify your configuration:
git config --list
Basic GitHub CLI Commands
Creating a Repository
You can create a new repository on GitHub using the following command:
gh repo create <repository-name> --public
<repository-name>
: The name of the new repository.--public
: Optional flag to make the repository public (default is private).
Example:
gh repo create my-new-repo --public
Cloning a Repository
To clone an existing repository to your local machine:
gh repo clone <owner>/<repository>
<owner>
: The GitHub username or organization that owns the repository.<repository>
: The name of the repository.
Example:
gh repo clone octocat/hello-world
Git Add
The git add
command adds changes in the working directory to the staging area.
Example:
- Make some changes to your files.
- Add the changes to the staging area:
git add .
Git Commit
The git commit
command saves your changes to the local repository.
Example:
git commit -m "Add new feature"
Pushing Changes
To push your local changes to the remote repository:
git push origin main
Pulling Changes
To pull changes from the remote repository to your local machine:
git pull origin main
Creating and Checking Out Branches
To create a new branch and switch to it:
- Create a new branch:
git branch <branch-name>
- Switch to the new branch
git checkout <branch-name>
Or do both in one step:
git checkout -b <branch-name>
Git Remote
To view the remote repositories:
git remote -v
To add a new remote repository:
git remote add origin <remote-url>
Managing Issues
Creating an Issue
To create a new issue:
gh issue create --title "Issue Title" --body "Issue description"
Example:
gh issue create --title "Bug in login feature" --body "There is a bug in the login feature that needs to be fixed."
Listing Issues
gh issue list
To list all issues in a repository:
gh repo view <owner>/<repository>
Viewing Repository Information
To view information about a repository:
Example:
gh repo view octocat/hello-world
Pull Request
A Pull Request (PR) is a fundamental feature in Git-based version control systems like GitHub, GitLab, and Bitbucket. It facilitates collaboration on projects by allowing developers to propose changes to a codebase and request that these changes be reviewed and integrated into the main codebase.
Key Concepts of a Pull Request
- Proposal of Changes:
- A PR is essentially a request to merge changes from one branch (often a feature branch) into another branch (usually the main or master branch). The branch with changes is known as the “source” branch, and the branch you want to merge into is the “target” branch.
- Code Review:
- Before changes are merged, team members or project maintainers review the proposed changes. This review process allows others to comment on the code, suggest improvements, and ensure that the changes meet the project’s standards and requirements.
- Discussion and Feedback:
- PRs provide a space for discussion around the changes. Reviewers can leave comments on specific lines of code or the overall changes. The author of the PR can respond to feedback, make updates, and push additional commits to address any concerns.
- Testing and Validation:
- Often, automated tests are run on the changes proposed in the PR to ensure that they do not introduce new bugs. Continuous Integration (CI) systems may automatically test the code to verify that it works correctly and does not break any existing functionality.
- Approval and Merging:
- Once the changes have been reviewed, discussed, and tested, and if there are no remaining issues, the PR can be approved and merged into the target branch. This process integrates the proposed changes into the main codebase.
- Closing a Pull Request:
- After the PR has been merged, or if it is no longer needed, it can be closed. This action signals that the proposed changes are either incorporated or discarded.
Example Workflow of a Pull Request
- Create a Feature Branch:
- A developer creates a new branch to work on a specific feature or fix a bug.
- Commit Changes:
- The developer makes changes and commits them to the feature branch.
- Push to Remote Repository:
- The developer pushes the feature branch to the remote repository (e.g., GitHub).
- Open a Pull Request:
- The developer opens a PR from the feature branch to the target branch (e.g.,
main
). They provide a title and description for the PR.
- The developer opens a PR from the feature branch to the target branch (e.g.,
- Review and Discuss:
- Team members review the PR, discuss the changes, and suggest improvements.
- Make Revisions:
- The developer addresses feedback by making additional changes and pushing them to the feature branch.
- Approve and Merge:
- Once the PR is approved and passes any automated tests, it is merged into the target branch.
- Close the Pull Request:
- After merging, the PR is closed, and the feature branch may be deleted if it is no longer needed.
Benefits of Pull Requests
- Collaboration: Encourages team members to collaborate on code changes.
- Quality Assurance: Ensures that code is reviewed and tested before integration.
- Documentation: Provides a record of changes, discussions, and decisions.
- Version Control: Maintains a clean and organized commit history.
Pull Requests are a vital part of modern software development workflows, promoting code quality, team collaboration, and project transparency.
Step-by-Step Guide to Making a Pull Request
1. Fork and Clone the Repository
If you’re contributing to a repository that you don’t have write access to, you’ll need to fork it first.
Using GitHub Website:
- Go to the repository you want to contribute to.
- Click on the “Fork” button in the upper right corner of the page.
Using GitHub CLI:
gh repo fork <repository> --clone=true
<repository>
: The repository you want to fork and clone.
Example:
gh repo fork octocat/hello-world --clone=true
2. Create a New Branch
Before making any changes, create a new branch to keep your modifications separate from the main branch.
git checkout -b <branch-name>
<branch-name>
: Name of your new branch.
Example:
git checkout -b feature/new-feature
3. Make Changes and Commit
Make your changes to the codebase. After making changes, you need to stage and commit them.
Stage Changes:
git add .
Commit Changes:
git commit -m "Add a detailed message about your changes"
4. Push Your Changes
Push your new branch and commits to your forked repository on GitHub.
git push origin <branch-name>
Example:
git push origin feature/new-feature
5. Create a Pull Request
Now, you need to create a Pull Request to propose your changes to the original repository.
Using GitHub CLI:
- Make sure you’re in the repository directory.
- Create a Pull Request with the following command:
gh pr create --base <base-branch> --head <branch-name> --title "Pull Request Title" --body "Description of the changes"
<base-branch>
: The branch you want to merge your changes into (typicallymain
ormaster
).<branch-name>
: The branch you created with your changes.--title
: The title of your Pull Request.--body
: A detailed description of your changes.
Example:
gh pr create --base main --head feature/new-feature --title "Add new feature" --body "This Pull Request adds a new feature to the application."
Using GitHub Website:
- Go to the original repository where you want to create the Pull Request.
- Click on the “Pull Requests” tab.
- Click on the “New Pull Request” button.
- Select the base branch (e.g.,
main
) and compare it with your feature branch. - Add a title and description for your Pull Request.
- Click “Create Pull Request.”
6. Review and Address Feedback
Once the Pull Request is created, it will be reviewed by the maintainers of the original repository. They might request changes or provide feedback.
- Check the PR conversation and review comments.
- Make any necessary changes and commit them to your branch.
- Push the updates to the same branch:
git push origin <branch-name>
7. Merge the Pull Request
If you have the permissions to merge, you can merge the Pull Request once it is approved.
Using GitHub Website:
- Go to the Pull Request page.
- Click on the “Merge pull request” button.
- Confirm the merge.
Using GitHub CLI (if you have permissions):
gh pr merge <pr-number>
<pr-number>
: The number of the Pull Request you want to merge.
Example:
gh pr merge 42
8. Delete the Branch (Optional)
After merging, you might want to delete the branch to keep the repository clean.
Using GitHub CLI:
gh pr close <pr-number> --delete-branch
Using GitHub Website:
- Go to the Pull Request page.
- Click on the “Delete branch” button.
Best Practices
- Consistent Naming: Use clear and consistent naming conventions for repositories, branches, and commit messages.
- Frequent Commits: Commit your changes frequently with meaningful commit messages.
- Use Issues and Pull Requests: Manage your work and collaborations using GitHub issues and pull requests.
- Branching Strategy: Adopt a branching strategy that suits your workflow (e.g., Git Flow, GitHub Flow).
Conclusion
Using GitHub CLI can significantly streamline your workflow by allowing you to perform GitHub operations directly from the command line. This guide covered the basics of setting up and using GitHub CLI for common tasks. As you become more comfortable with these commands, you can explore more advanced features of the CLI to enhance your productivity.
Feel free to ask if you have any specific questions or need further assistance with GitHub CLI!