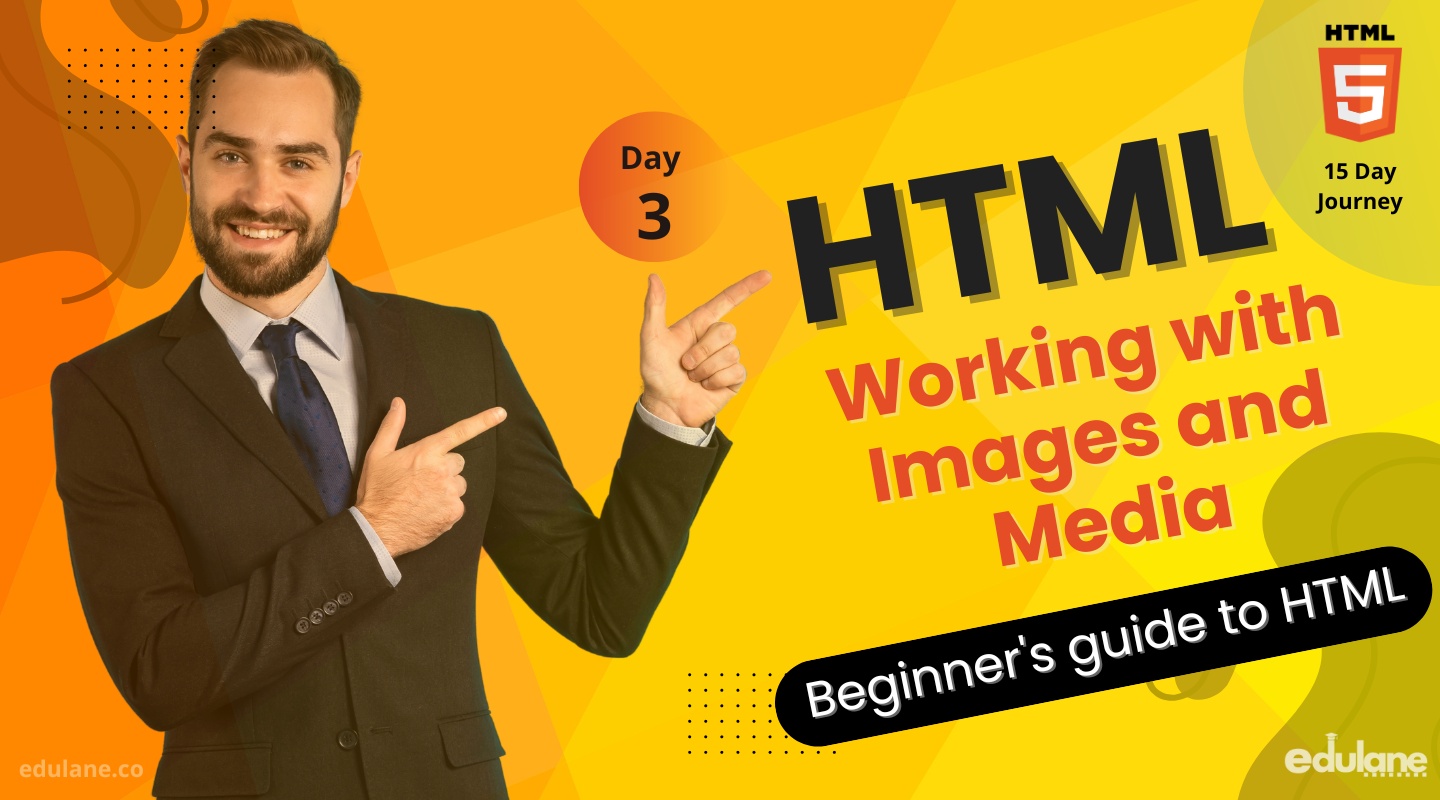
Mastering Media Integration: Embedding Images, Videos, and Audio in HTML
In this session, you will learn how to enhance your HTML pages with various forms of media, including images, videos, and audio. We will cover the following key topics:
- Embedding Images: Discover how to use the
<img>
tag to include images in your HTML documents. Learn about essential attributes likesrc
for specifying the image source,alt
for providing alternative text, andwidth
andheight
for controlling the image size. Understand best practices for optimizing images to improve page load times and maintain visual quality. - Adding Videos and Audio: Explore how to embed multimedia content using HTML5’s
<video>
and<audio>
tags. Learn to add playback controls for user interaction and handle various media formats. This section will help you integrate rich media content seamlessly into your web pages. - Responsive Images: Delve into techniques for making images responsive to different screen sizes using the
srcset
attribute and the<picture>
element. These methods ensure that your images look great on all devices, from mobile phones to desktop monitors.
By the end of this session, you will have a solid understanding of how to incorporate and manage media content in your HTML projects, making your web pages more engaging and interactive.
Table of Contents
- Embedding Images
- Adding Videos and Audio
- Responsive Images
- Exercise
- Git Commit Process
- Real-Time Media Content Examples
What is Embedding Images?
Explanation: Embedding images involves inserting image files into your web pages so that they are displayed as part of your content. This process allows you to enrich your web pages with visual elements, which can enhance user engagement, illustrate content, and support your messaging.
How it Works:
- HTML
<img>
Tag: The primary way to embed images in HTML is by using the<img>
tag. This tag points to the image file through itssrc
attribute and provides options for defining the image’s size and alternate text. - Image File Location: The image file can be located either on the same server as your website (relative path) or hosted externally (absolute URL).
Key Components:
- Source Attribute (
src
): This attribute specifies the location of the image file. The browser uses this URL to fetch and display the image on the page.- Example:
src="https://example.com/path/to/image.jpg"
- Example:
- Alternative Text Attribute (
alt
): Provides a text description of the image. This text is displayed if the image cannot be loaded and is used by screen readers to describe the image to visually impaired users.- Example:
alt="A scenic view of mountains at sunset"
- Example:
- Dimensions (
width
andheight
): These optional attributes set the image’s display size in pixels. Specifying dimensions helps control layout and maintain consistency across different devices.- Example:
width="600" height="400"
- Example:
Why Embedding Images is Important:
- Visual Appeal: Images make web pages more engaging and visually appealing, breaking up text-heavy content and attracting users’ attention.
- Content Enhancement: They can illustrate concepts, provide context, and enhance the overall communication of your message.
- User Experience: Properly embedded and optimized images contribute to a better user experience by ensuring that images load quickly and display correctly on various devices.
Best Practices:
- Optimize Images: Compress images to reduce file size without sacrificing quality. This helps improve page load times and performance.
- Use Appropriate Formats: Choose the right image format based on the image content:
- JPEG: For photographs and images with complex colors.
- PNG: For images with transparency or sharp lines.
- SVG: For scalable vector graphics like icons and logos.
- Responsive Images: Implement responsive design techniques, such as using the
srcset
attribute or the<picture>
element, to ensure images adapt to different screen sizes and resolutions.
What is Using the <img> Tag?
Explanation: The <img>
tag is an HTML element used to embed images into your web pages. It allows you to display visual content by referencing an image file through its source URL. This tag is essential for adding pictures, illustrations, and other graphical elements to your website.
Key Points:
- Self-Closing Tag: The
<img>
tag is self-closing, meaning it doesn’t have a closing counterpart like many other HTML tags. - Attributes: The tag requires the
src
attribute to specify the image location and thealt
attribute to describe the image for accessibility.
Example:
<img src="https://example.com/sample.jpg" alt="A beautiful sunset" width="600" height="400">
This code embeds an image from the specified URL, with alternative text provided and dimensions set for display.
What are Image Attributes?
Explanation: Image attributes are specific properties of the <img>
tag that control how an image is displayed on a webpage. These attributes help define the image source, alternative text, and dimensions, ensuring proper rendering and accessibility.
Key Points:
src
(Source): Specifies the path to the image file. This can be a relative path or an absolute URL. It is essential for the image to appear on the page.alt
(Alternative Text): Provides a textual description of the image. This is crucial for accessibility and SEO, as it describes the image content to users who cannot see it.width
andheight
: Set the dimensions of the image in pixels. While optional, these attributes help maintain layout consistency and control the image’s display size.
<img src="https://example.com/sample1.jpg" alt="Sample Image 1" width="300" height="200">
<img src="https://example.com/sample2.jpg" alt="Sample Image 2">
What are Best Practices for Images?
Explanation: Best practices for images involve techniques and strategies to optimize their performance and quality on web pages. Proper image handling improves load times, visual appeal, and overall user experience.
Key Points:
- File Formats: Choose the right format for your images:
- JPEG: Ideal for photographs and images with gradients.
- PNG: Best for images that require transparency.
- SVG: Suitable for vector graphics and icons.
- Compression: Reduce the image file size without significantly affecting quality. Use tools like TinyPNG or ImageOptim for compression.
- Responsive Images: Use techniques such as the
srcset
attribute and the<picture>
element to ensure images adapt to different screen sizes and resolutions. - Accessibility: Always provide meaningful
alt
text to describe the image content for users with visual impairments and to enhance search engine optimization.
Example:
<picture>
<source srcset="https://example.com/image-small.jpg" media="(max-width: 600px)">
<source srcset="https://example.com/image-medium.jpg" media="(max-width: 1200px)">
<img src="https://example.com/image-large.jpg" alt="High-quality image of a sunset" width="800" height="600">
</picture>
This code demonstrates how to use the <picture>
element and srcset
attribute for responsive image handling.
By understanding these key concepts and applying best practices, you can effectively embed and manage images on your web pages, ensuring they are visually appealing, functional, and accessible.
Project: Personal Portfolio Gallery
Create a personal portfolio webpage that displays an image gallery with embedded images. The gallery will use both relative and absolute paths, demonstrate image optimization practices, and include responsive images for different screen sizes.
Project Outline:
- Setup the Project Structure:
- Create a project directory with the following structure:
portfolio-gallery/
├── images/
│ ├── local-image1.jpg
│ ├── local-image2.jpg
├── index.html
- Create
index.html
:- This HTML file will include the image gallery with examples of relative and absolute paths, and responsive images.
- Add Images:
- Place the images
local-image1.jpg
andlocal-image2.jpg
in theimages
folder. - For demonstration, use an absolute URL for an additional image hosted online.
- Place the images
HTML Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Personal Portfolio Gallery</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
.gallery {
display: flex;
flex-wrap: wrap;
justify-content: center;
}
.gallery img {
margin: 10px;
border: 2px solid #ddd;
border-radius: 8px;
width: 300px;
height: auto;
}
</style>
</head>
<body>
<h1>My Portfolio Gallery</h1>
<p>Welcome to my portfolio! Here are some of my favorite images:</p>
<div class="gallery">
<!-- Relative Path Image -->
<img src="images/local-image1.jpg" alt="Local Image 1" width="300" height="200">
<!-- Another Relative Path Image -->
<img src="images/local-image2.jpg" alt="Local Image 2" width="300" height="200">
<!-- Absolute URL Image -->
<img src="https://example.com/images/external-image.jpg" alt="External Image" width="300" height="200">
<!-- Responsive Image -->
<picture>
<source srcset="https://example.com/images/small.jpg" media="(max-width: 600px)">
<source srcset="https://example.com/images/medium.jpg" media="(max-width: 1200px)">
<img src="https://example.com/images/large.jpg" alt="Responsive Image" width="300" height="200">
</picture>
</div>
</body>
</html>
Explanation:
- HTML Structure:
<head>
Section: Contains meta tags for character set and viewport, a title for the page, and inline CSS for styling.<body>
Section: Includes a heading and a paragraph introducing the gallery.
- Image Embedding:
- Relative Path Images:
local-image1.jpg
andlocal-image2.jpg
are referenced using relative paths. They are stored in theimages
folder within the project directory. - Absolute URL Image: An image hosted on an external server is included using an absolute URL.
- Responsive Image: The
<picture>
element is used to provide different image sources based on screen width for better responsiveness.
- Relative Path Images:
- Styling:
- Gallery Container: The
.gallery
class uses Flexbox to arrange images in a flexible grid. - Image Styling: Images have margins, borders, and rounded corners for a polished look.
- Gallery Container: The
This project demonstrates embedding images using both relative and absolute paths, implements best practices for image optimization, and showcases responsive design techniques. By following this example, you can create a visually appealing and functional image gallery for any web project.
Adding Videos and Audio
Explanation: Embedding videos and audio files allows you to enrich your web pages with multimedia content. This can include promotional videos, tutorials, background music, and more. HTML5 provides native support for embedding these media types using the <video>
and <audio>
tags.
Embedding Videos
Explanation: The <video>
tag is used to embed video files on a web page. This tag supports multiple video formats and provides various attributes to control playback, such as controls, autoplay, and loop.
Key Attributes:
src
: Specifies the URL of the video file.- Example:
src="video.mp4"
- Example:
controls
: Adds video controls like play, pause, and volume.- Example:
controls
- Example:
autoplay
: Starts playing the video automatically when the page loads.- Example:
autoplay
- Example:
loop
: Repeats the video indefinitely.- Example:
loop
- Example:
poster
: Displays an image before the video starts playing.- Example:
poster="thumbnail.jpg"
- Example:
Example of Embedding a Video:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Embedding Videos Example</title>
</head>
<body>
<h1>Video Example</h1>
<video width="640" height="360" controls autoplay>
<source src="video.mp4" type="video/mp4">
<source src="video.ogg" type="video/ogg">
Your browser does not support the video tag.
</video>
</body>
</html>
Explanation:
<video>
Tag: Embeds the video file on the page.controls
Attribute: Provides playback controls.autoplay
Attribute: Starts the video automatically.<source>
Tags: Allow for multiple video formats to ensure compatibility with different browsers.- Fallback Message: Displays a message if the video tag is not supported.
Embedding Audio
Explanation: The <audio>
tag is used to embed audio files. It provides attributes similar to the <video>
tag for controlling playback.
Key Attributes:
src
: Specifies the URL of the audio file.- Example:
src="audio.mp3"
- Example:
controls
: Adds audio controls such as play, pause, and volume.- Example:
controls
- Example:
autoplay
: Plays the audio automatically when the page loads.- Example:
autoplay
- Example:
loop
: Repeats the audio indefinitely.- Example:
loop
- Example:
Example of Embedding Audio:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Embedding Audio Example</title>
</head>
<body>
<h1>Audio Example</h1>
<audio controls autoplay>
<source src="audio.mp3" type="audio/mp3">
<source src="audio.ogg" type="audio/ogg">
Your browser does not support the audio element.
</audio>
</body>
</html>
Explanation:
<audio>
Tag: Embeds the audio file on the page.controls
Attribute: Provides audio playback controls.autoplay
Attribute: Starts the audio automatically.<source>
Tags: Allow for multiple audio formats to ensure compatibility with different browsers.- Fallback Message: Displays a message if the audio element is not supported.
Best Practices for Embedding Videos and Audio:
- Format Compatibility: Use multiple formats for better cross-browser compatibility.
- Video Formats: MP4, WebM, Ogg
- Audio Formats: MP3, Ogg
- Optimize Files: Compress videos and audio to reduce file size and improve loading times.
- Provide Controls: Ensure users have control over playback, such as play, pause, and volume.
By incorporating these techniques, you can effectively add video and audio content to your web pages, enhancing user experience and providing richer media experiences.
Responsive Images
Explanation: Responsive images are designed to adapt to different screen sizes and resolutions. This ensures that images look good and load efficiently on all devices, from mobile phones to large desktop monitors. Using responsive techniques helps improve page load times and user experience by serving appropriately sized images based on the device’s viewport.
Using srcset
Explanation: The srcset
attribute of the <img>
tag allows you to specify different image sources for different screen resolutions or viewport widths. This enables the browser to choose the most suitable image based on the device’s display characteristics.
How It Works:
srcset
Attribute: Lists multiple image sources along with their corresponding sizes or resolutions.sizes
Attribute (optional): Defines the image display width in different viewport widths, helping the browser select the most appropriate image fromsrcset
.
Example of Using srcset
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Images with srcset</title>
</head>
<body>
<h1>Responsive Image Example</h1>
<img src="small.jpg"
srcset="small.jpg 480w,
medium.jpg 800w,
large.jpg 1200w"
sizes="(max-width: 600px) 480px,
(max-width: 1200px) 800px,
1200px"
alt="A responsive image">
</body>
</html>
Explanation:
srcset
Attribute: Defines three image sources with different widths (480w, 800w, 1200w). The browser chooses the image based on the viewport width.sizes
Attribute: Specifies how the image should be sized in different viewport conditions. For example, if the viewport is up to 600px wide, the browser will use the 480px image.
Using the <picture>
Element
Explanation: The <picture>
element provides more control over which images are loaded based on media queries and other conditions. It allows you to specify different images for different screen sizes and resolutions, and even different image formats.
How It Works:
<picture>
Element: Contains one or more<source>
elements and a fallback<img>
element.<source>
Element: Each source specifies an image file and a media query or type attribute to select when to use that source.
Example of Using the <picture>
Element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Images with Picture Element</title>
</head>
<body>
<h1>Responsive Picture Example</h1>
<picture>
<source srcset="small.jpg" media="(max-width: 600px)">
<source srcset="medium.jpg" media="(max-width: 1200px)">
<img src="large.jpg" alt="A responsive image using picture element">
</picture>
</body>
</html>
Explanation:
<picture>
Element: Wraps multiple<source>
elements and a fallback<img>
element.<source>
Elements: Each<source>
specifies an image file and a media condition. For instance,small.jpg
is used for viewports up to 600px wide, andmedium.jpg
for viewports up to 1200px.- Fallback
<img>
Element: Provides a default image if none of the<source>
conditions are met or if the browser does not support the<picture>
element.
Best Practices for Responsive Images:
- Use Appropriate Image Formats: Choose formats that balance quality and file size.
- JPEG: For photos and images with many colors.
- PNG: For images requiring transparency or sharp details.
- WebP: For high-quality compression with smaller file sizes.
- Optimize Images: Compress images to reduce file size while maintaining acceptable quality.
- Test Across Devices: Ensure images display correctly on various devices and screen sizes.
By implementing srcset
and the <picture>
element, you can provide an optimal viewing experience for users by serving appropriately sized images based on their device’s capabilities.
Exercise: Multimedia and Responsive Design
Objective:
Create a webpage that demonstrates embedding images, videos, and audio, as well as implementing responsive image techniques using srcset
and the <picture>
element.
Project Structure:
- Project Directory:
multimedia-exercise/
├── images/
│ ├── example1.jpg
│ ├── example2.jpg
│ ├── small.jpg
│ ├── medium.jpg
│ ├── large.jpg
├── video/
│ ├── sample.mp4
│ ├── sample.ogg
├── audio/
│ ├── background.mp3
│ ├── background.ogg
├── index.html
HTML Code for index.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Multimedia and Responsive Design</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 0;
padding: 0;
background-color: #f4f4f4;
}
.container {
max-width: 1200px;
margin: auto;
padding: 20px;
}
.gallery img, .media video, .media audio {
margin: 10px;
border: 2px solid #ddd;
border-radius: 8px;
max-width: 100%;
height: auto;
}
</style>
</head>
<body>
<div class="container">
<h1>Multimedia and Responsive Design Exercise</h1>
<!-- Embedding Images -->
<section>
<h2>1. Embedding Images</h2>
<p>Below are examples of images embedded using both relative and absolute paths:</p>
<!-- Relative Path Image -->
<img src="images/example1.jpg" alt="Example Image 1" width="400" height="300">
<!-- Comment: This image is sourced from the local 'images' directory using a relative path. -->
<!-- Absolute URL Image -->
<img src="https://via.placeholder.com/400x300" alt="Placeholder Image" width="400" height="300">
<!-- Comment: This image is sourced from an external URL. -->
</section>
<!-- Embedding Videos -->
<section class="media">
<h2>2. Embedding Videos</h2>
<p>Below is an example of a video embedded using the <code><video></code> tag:</p>
<video width="640" height="360" controls>
<source src="video/sample.mp4" type="video/mp4">
<source src="video/sample.ogg" type="video/ogg">
<!-- Comment: Multiple video formats are provided for better compatibility. -->
Your browser does not support the video tag.
</video>
</section>
<!-- Embedding Audio -->
<section class="media">
<h2>3. Embedding Audio</h2>
<p>Below is an example of audio embedded using the <code><audio></code> tag:</p>
<audio controls>
<source src="audio/background.mp3" type="audio/mp3">
<source src="audio/background.ogg" type="audio/ogg">
<!-- Comment: Multiple audio formats are provided for better compatibility. -->
Your browser does not support the audio element.
</audio>
</section>
<!-- Responsive Images -->
<section>
<h2>4. Responsive Images</h2>
<p>Below are examples of responsive images using <code>srcset</code> and the <code><picture></code> element:</p>
<!-- Responsive Image with srcset -->
<img src="images/small.jpg"
srcset="images/small.jpg 480w,
images/medium.jpg 800w,
images/large.jpg 1200w"
sizes="(max-width: 600px) 480px,
(max-width: 1200px) 800px,
1200px"
alt="Responsive Image with srcset">
<!-- Comment: The srcset attribute provides different image sources based on viewport width. -->
<!-- Responsive Image with <picture> -->
<picture>
<source srcset="images/small.jpg" media="(max-width: 600px)">
<source srcset="images/medium.jpg" media="(max-width: 1200px)">
<img src="images/large.jpg" alt="Responsive Image with Picture Element">
<!-- Comment: The <picture> element selects different images based on media queries. -->
</picture>
</section>
</div>
</body>
</html>
Instructions for the Exercise:
- Embedding Images:
- Use the
<img>
tag to embed an image from theimages
folder using a relative path. - Add another image using an absolute URL to demonstrate linking to external resources.
- Use the
- Embedding Videos:
- Use the
<video>
tag to include a video file. Provide multiple video sources for different formats (MP4 and Ogg).
- Use the
- Embedding Audio:
- Use the
<audio>
tag to include an audio file. Provide multiple audio sources for different formats (MP3 and Ogg).
- Use the
- Responsive Images:
- Implement responsive images using the
srcset
attribute in the<img>
tag. - Use the
<picture>
element to show how different images can be served based on viewport conditions.
- Implement responsive images using the
Git Commit Process
Overview: Committing changes to Git involves recording modifications to files in your local repository. Proper commit messages and organized commits help maintain a clear project history.
Steps for Committing Changes:
- Initialize Git Repository (if not already initialized):
git init
Explanation: Initializes a new Git repository in the current directory.
- Check the Status of Your Files:
git status
Explanation: Displays the status of changes in your working directory and staging area. This will show you which files are modified and need to be staged for committing.
- Stage Files for Commit:
git add index.html
git add images/
git add video/
git add audio/
Explanation: Stages the specified files and directories for committing. You can stage all modified files by using git add .
, but specifying files explicitly helps in making precise commits.
- Commit Your Changes:
git commit -m "Add multimedia and responsive design examples"
Explanation: Commits the staged changes with a descriptive message. The -m
flag allows you to write a commit message directly from the command line.
- Review Commit History:
git log
Explanation: Displays the commit history for your repository. Each commit will have a unique SHA-1 hash, author information, date, and commit message.
- Push Changes to Remote Repository (if applicable):
git push origin main
Explanation: Pushes your commits to a remote repository (e.g., GitHub, GitLab). Replace main
with the branch name you are working on.
Commit Message Guidelines:
- Be Descriptive: Write a clear, concise message that explains what changes were made. Avoid generic messages like “Update files” or “Changes.”Example:
Add multimedia elements and responsive images examples
- Embedded images using relative and absolute paths.
- Added video and audio examples with multiple formats.
- Implemented responsive images using srcset and <picture> element.
- Use Present Tense: Describe the changes in present tense, as if you are explaining what the commit does.
Example: “Fixes layout issue in the responsive image section.”
- Include Context (if necessary): If the commit relates to a specific issue or feature, mention it in the commit message.
Example: “Fixes bug #123: Corrected paths for video files.”