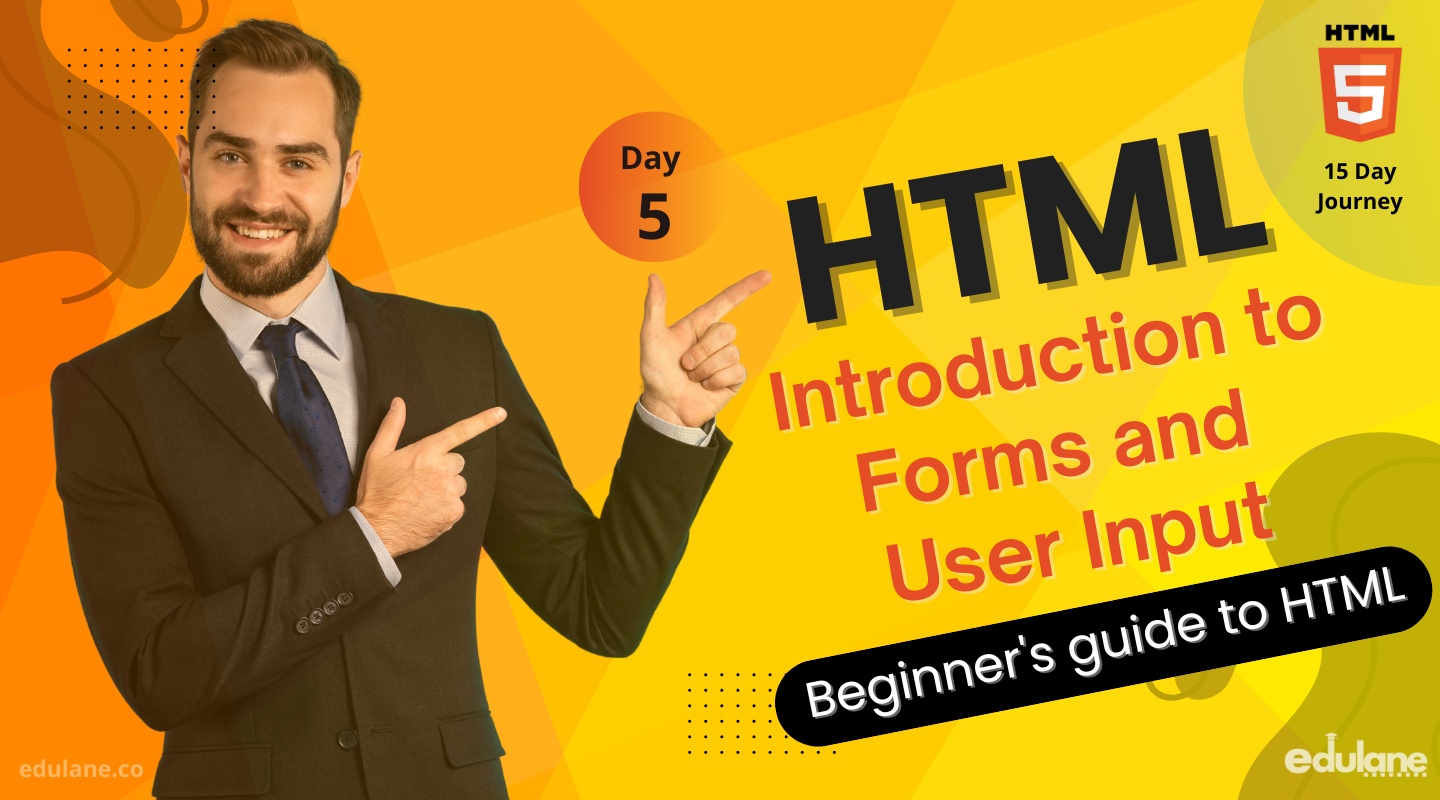
Building Interactive Web Forms: A Comprehensive Guide to HTML Forms and User Input
In today’s digital world, user interaction on websites is driven by forms. Whether you are signing up for a newsletter, logging into an account, or submitting feedback, forms are the key component that facilitates communication between the user and the server. Understanding how to build and manage HTML forms is essential for any web developer, as they are one of the most common ways to collect user input and send data to servers.
This session will guide you through the step-by-step process of creating interactive forms that not only look good but also function effectively. You will start by learning the basic structure of an HTML form using core elements like <form>
, <input>
, <label>
, and move on to organizing fields with <fieldset>
and <legend>
. You will then explore the various types of input fields available, such as text, password, email, and submit buttons, and understand how to implement them based on specific use cases.
Moreover, you will dive into how forms communicate with servers using the action
and method
attributes, an essential part of any form’s functionality. By the end of this session, you will have the knowledge to not only design a user-friendly form but also understand how it operates behind the scenes by sending data to a web server.
Why This Session Is Important:
Web forms are used in almost every web application for a variety of purposes—sign-ups, logins, contact forms, surveys, and more. Understanding how to build accessible, secure, and functional forms is crucial for creating interactive, data-driven web applications. Mastering forms ensures you are capable of building the foundational aspects of user interaction in websites and applications, giving you the ability to process user input and improve user experience.
Key Learning Outcomes:
By the end of this session, candidates will be able to:
- Understand the structure of HTML forms: Learn how to use the
<form>
,<input>
,<label>
, and other related elements. - Implement common input types: Master input fields like text, password, email, and the submit button, and understand when and why to use each.
- Organize form data: Group form fields logically using
<fieldset>
and<legend>
for better readability and accessibility. - Send form data to servers: Gain practical knowledge about the
action
andmethod
attributes to control how and where form data is sent. - Create a fully functional contact form: Apply the knowledge gained to build a contact form as part of the project, which will be a practical, real-world application of the skills learned.
What You Will Learn:
- Form Structure
Learn how to create and structure HTML forms using the<form>
,<input>
, and<label>
tags. Understand how to collect user input in various formats. - Input Types
Explore the different input types available in HTML, such as text fields, password fields, email fields, and buttons. Learn how to implement and validate these fields to ensure accurate user input. - Form Attributes
Understand how theaction
andmethod
attributes control how form data is sent to the server, and when to use each one (e.g.,GET
vs.POST
methods). - Form Grouping and Accessibility
Use<fieldset>
and<legend>
to group form fields together in a way that improves user experience and makes your form more accessible for everyone. - Form Project: Building a Contact Form
Get hands-on experience by building a contact form from scratch that includes various input types like text, email, and message fields, along with a submit button. This project will give you practical exposure to working with forms and handling user input.
Why This Is Important for Web Developers:
Forms are a vital part of any website or web application because they allow the collection and submission of data. Whether it’s capturing user information, taking orders, or managing feedback, forms are the backbone of user interaction.
By mastering HTML forms, you will be able to:
- Develop user-friendly websites that provide clear, concise, and organized ways for users to interact with your application.
- Create accessible and secure forms, ensuring data is correctly validated before submission and sent securely to the server.
- Implement best practices in form design, improving the overall user experience and ensuring the forms are intuitive and easy to navigate.
Skills Acquired:
- Creating forms with various input types.
- Implementing accessible labels and organizing forms with
<fieldset>
and<legend>
. - Validating user input using required fields and input constraints.
- Understanding server-side form submission and form security considerations.
- Building and testing real-world web forms.
This session forms the cornerstone of learning how to collect, validate, and process data on the web. By the end of the session, candidates will have developed a fully functional contact form, ready for real-world use, and have the foundational knowledge to create more complex forms in the future.
Meta Data for Day 5: Building Interactive Web Forms
- Title: Building Interactive Web Forms: A Comprehensive Guide to HTML Forms and User Input
- Description: In this session, you will learn how to create and structure HTML forms, explore various input types such as text, password, email, and submit buttons, and understand how forms interact with servers using the
action
andmethod
attributes. By the end, you’ll build a functional contact form and gain the knowledge to handle user input effectively. - Keywords: HTML forms, user input, web development, form attributes, input types, form submission, action and method, contact form project,
<form>
,<input>
,<label>
,<fieldset>
, web forms, form validation - Duration: 1.5 hours
- Skill Level: Beginner to Intermediate
- Audience: Aspiring web developers, frontend developers, HTML beginners
- Prerequisites: Basic knowledge of HTML structure
- Learning Objectives:
- Understand the basic structure of HTML forms.
- Implement different input types to collect user data.
- Use form attributes like
action
andmethod
to handle form submission. - Build a real-world project by creating a contact form.
- Instructor: Sunil Sharama
- Tags: HTML, Web Forms, Input Types, Data Collection, Form Submission, Web Development Best Practices, Contact Form
Table of Contents
- Session Preview
- Session Objectives
- Session Details
3.1. Form Basics
– The<form>
Element
– The<input>
Element
– The<label>
Element
– The<fieldset>
and<legend>
Elements
3.2. Common Input Types
– Text Input
– Password Input
– Email Input
– Submit Button
3.3. Form Action and Method
– Theaction
Attribute
– Themethod
Attribute - Project: Build a Contact Form
4.1. Project Goals
4.2. Instructions
4.3. Code Example - Interview Questions
Session Details
3.1. Form Basics
In this section, we will cover the foundational elements required to build a functional HTML form. These elements provide the basic structure that allows users to input and submit data on websites.
The <form>
Element
The <form>
tag is the core container for any HTML form. It wraps all form controls such as text fields, checkboxes, radio buttons, submit buttons, and more. Without a <form>
tag, the input elements on a page would not function as a cohesive unit to submit data.
- Attributes:
action
: Specifies the URL where the form data will be submitted.method
: Defines how the form data is sent to the server (eitherGET
orPOST
).enctype
: Used for file uploads, it defines the encoding type of the form data when sent to the server. (e.g.,multipart/form-data
)
Example:
<form action="/submit-form" method="post">
<!-- Form elements go here -->
</form>
In this example, the form will submit its data to the /submit-form
URL using the POST
method.
The <input>
Element
The <input>
tag represents various types of input fields, allowing users to enter different kinds of data. It is a self-closing tag and is one of the most flexible elements in HTML, as it can be adapted to collect text, passwords, emails, numbers, and more.
- Attributes:
type
: Defines the type of input field (e.g.,text
,email
,password
,checkbox
,radio
, etc.).name
: The name attribute identifies the input data when it’s submitted to the server.id
: Uniquely identifies the input field, which is used to link with a<label>
.placeholder
: Provides a hint to the user about what type of input is expected.required
: Makes the field mandatory before form submission.
Example:
<input type="text" name="username" id="username" placeholder="Enter your username" required>
Here, the input is a text field with the placeholder “Enter your username,” and it is required for submission.
The <label>
Element
The <label>
tag is used to define labels for form controls, particularly input fields. Using labels improves form accessibility, as it allows users (especially those using screen readers) to understand what each input field is for. When a label is associated with an input field, clicking the label will focus the corresponding input.
- Attributes:
for
: Connects the label to a specific input field by referencing the input’sid
.
Example:
<label for="email">Email:</label>
<input type="email" id="email" name="email" placeholder="Enter your email" required>
In this example, the label “Email” is associated with the email input field. Clicking the label will focus on the email field.
The <fieldset>
and <legend>
Elements
The <fieldset>
element is used to group related form controls into a section. This helps to organize complex forms, making them more readable and user-friendly. Inside a <fieldset>
, you can use the <legend>
element to provide a caption or heading for the group of controls, giving users a clear understanding of the section’s purpose.
- The
<fieldset>
element groups related inputs. - The
<legend>
element provides a title or description for the grouped inputs.
Example:
<form>
<fieldset>
<legend>Personal Information</legend>
<label for="name">Name:</label>
<input type="text" id="name" name="name" required><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
</fieldset>
</form>
In this example, the <fieldset>
groups the name and email inputs under the “Personal Information” section, with the <legend>
acting as the section’s title.
3.2. Common Input Types
In this section, we will explore some of the most commonly used HTML input types. Each input type serves a specific purpose in forms and enables the collection of different kinds of data from users.
Text Input
The text input field is the most basic and commonly used input type in HTML. It allows users to enter single-line, freeform text. This input type is ideal for collecting short pieces of information such as names, usernames, or titles.
- Attributes:
type="text"
: Defines the input as a text field.name
: Specifies the name of the input field that will be sent to the server when the form is submitted.placeholder
: Provides a hint to the user about the expected content in the field.maxlength
: Limits the number of characters the user can input.required
: Ensures the field must be filled out before submitting the form.
Example:
<label for="name">Name:</label>
<input type="text" id="name" name="name" placeholder="Enter your name" required>
In this example, the user is prompted to enter their name. The required
attribute makes sure that the form cannot be submitted unless this field is filled out.
Password Input
The password input field allows users to enter sensitive data such as passwords. Unlike a regular text input, characters in a password field are obscured by dots or asterisks to prevent others from seeing what is being typed.
- Attributes:
type="password"
: Defines the input as a password field, ensuring that characters are hidden.name
: Identifies the password field when the form data is sent to the server.maxlength
: Sets the maximum number of characters the user can enter.required
: Makes this field mandatory for form submission.
Example:
<label for="password">Password:</label>
<input type="password" id="password" name="password" placeholder="Enter your password" required>
In this example, the user enters a password into a field where the characters are masked for security. The field is also required before the form can be submitted.
Email Input
The email input field is designed to accept email addresses and comes with built-in validation. It ensures that the value entered by the user follows the standard email format (e.g., user@example.com
). This makes it easier to validate email addresses before submitting the form data.
- Attributes:
type="email"
: Defines the input as an email field, enabling basic email validation.name
: Identifies the input field when submitting the form data.placeholder
: Provides a hint that informs the user to enter an email address.required
: Ensures the field is filled out before the form is submitted.
Example:
<label for="email">Email:</label>
<input type="email" id="email" name="email" placeholder="Enter your email address" required>
In this example, the user is prompted to enter a valid email address. The field will not accept non-email formats and requires proper email syntax (e.g., including “@” and a domain).
Submit Button
The submit button is used to send the form data to the server. Once the user has filled out the form, clicking the submit button triggers the form’s action
attribute to send the data, based on the method
specified (e.g., POST
or GET
).
- Attributes:
type="submit"
: Defines the button as a submit button.value
: The text displayed on the button.
Example:
<input type="submit" value="Submit">
In this example, the submit button sends the form data to the server when clicked. The value
attribute allows you to customize the text that appears on the button (in this case, “Submit”).
3.3. Form Action and Method
In this section, we will focus on how forms communicate with the server using the action
and method
attributes. These attributes are crucial for controlling how and where the form data is sent once the user submits the form.
The action
Attribute
The action
attribute in the <form>
element specifies the URL where the form data should be submitted. When the user fills out the form and clicks the submit button, the browser sends the form data to the server at the URL defined in the action
attribute. If the action
attribute is omitted, the form data is submitted to the current page’s URL.
- Purpose: Defines where the form data should be sent for processing.
- Format: The value of the
action
attribute is typically a URL (either an absolute or relative path). - Example Use Case: Submitting data to a server-side script, such as PHP or Python, that processes the form input.
Example:
<form action="/submit-form" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br><br>
<input type="submit" value="Submit">
</form>
In this example:
- The form data (username and email) will be submitted to
/submit-form
. - This path could point to a server-side script that processes the input.
If no action
attribute is provided, the form will submit to the current page URL, refreshing the page and sending data to itself.
The method
Attribute
The method
attribute specifies how the form data should be sent to the server. It determines the HTTP request method used when submitting the form. There are two common methods: GET
and POST
.
GET
Method:- Sends the form data as part of the URL in the query string.
- Best for retrieving data (e.g., search forms) or when the data is not sensitive.
- The data is visible in the URL and limited by the URL length (depending on the browser, usually around 2000 characters).
POST
Method:- Sends the form data as part of the HTTP request body, not as part of the URL.
- Best for sending sensitive or large amounts of data (e.g., login forms, registration forms).
- More secure than
GET
as the data is not visible in the URL, and there are no size limitations on the form data.
Example:
<form action="/submit-form" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br><br>
<input type="submit" value="Submit">
</form>
In this example:
- The form uses the
POST
method, meaning the data will be sent in the HTTP request body rather than appended to the URL. - This method is more secure and is commonly used for forms that send personal information (like login forms or forms with sensitive data).
When to Use GET
vs. POST
- Use
GET
when:- You are retrieving or querying information, such as in a search form.
- The form data is not sensitive.
- You want the data to be visible in the URL (e.g., for bookmarking or sharing).
Example:
<form action="/search" method="get">
<label for="query">Search:</label>
<input type="text" id="query" name="query" placeholder="Enter search term" required>
<input type="submit" value="Search">
</form>
In this case, the search query is visible in the URL after submission (/search?query=something
).
- Use
POST
when:- You are submitting data that modifies or creates resources on the server (e.g., registration forms, file uploads).
- The data is sensitive or large (e.g., passwords, personal details).
- You want to keep the form data hidden from the URL for security reasons.
Example:
<form action="/register" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required><br><br>
<input type="submit" value="Register">
</form>
Here, the form submits the username and password using the POST
method, which ensures the data remains secure during transmission.
Key Differences Between GET
and POST
:
Attribute | GET | POST |
---|---|---|
Data Location | Appended to URL in query string | Sent in HTTP request body |
Security | Less secure (data visible in URL) | More secure (data hidden from URL) |
Data Size | Limited by URL length | No size limit for data |
Use Case | Best for fetching or querying data | Best for sending sensitive data |
Project: Build a Contact Form
In this project, you will apply the knowledge gained from previous sections to build a fully functional contact form. The goal is to create a simple form that collects a user’s name, email, and a message, then submits this data to the server.
4.1. Project Goals
The main goals of this project are:
- Create a well-structured form: Utilize the
<form>
,<label>
, and<input>
tags to structure a contact form. - Use common input types: Implement different input types such as text, email, and textarea to gather various kinds of data.
- Ensure accessibility and usability: Use
<label>
and proper attributes to ensure the form is user-friendly and accessible. - Form submission: Use the
action
andmethod
attributes to define where the data will be sent and how it will be transmitted to the server.
4.2. Instructions
Follow these steps to build your contact form:
- Create the HTML structure:
- Start by creating a new HTML file, for example,
contact_form.html
. - Add a basic structure for the document using the
<html>
,<head>
, and<body>
tags.
- Start by creating a new HTML file, for example,
- Define the form:
- Use the
<form>
element to wrap all your input fields. - Set the
action
attribute to a URL where the form data will be submitted (for this project, you can use a dummy URL like"/submit-form"
). - Set the
method
attribute toPOST
, as we are simulating the submission of sensitive data.
- Use the
- Add form fields:
- Create three fields for collecting the user’s name, email, and message:
- Use the
text
input type for the user’s name. - Use the
email
input type for the user’s email with proper validation. - Use a
<textarea>
for the message input to allow multi-line input.
- Use the
- Ensure all fields are required using the
required
attribute.
- Create three fields for collecting the user’s name, email, and message:
- Add labels for accessibility:
- Each input field should have an associated
<label>
to describe the input, making the form more accessible for users with assistive technologies.
- Each input field should have an associated
- Include a submit button:
- Add a submit button to allow the user to submit the form data.
- Test the form:
- Open the form in your browser and fill it out to ensure the layout is user-friendly and functional.
- Although this project uses a dummy action URL, you can test form submission by pointing it to a real URL later on in your web development journey.
4.3. Code Example
Here is the complete HTML code for the contact form:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Contact Form</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
form {
max-width: 500px;
margin: auto;
}
label {
display: block;
margin-top: 10px;
}
input, textarea {
width: 100%;
padding: 8px;
margin-top: 5px;
margin-bottom: 15px;
border: 1px solid #ccc;
border-radius: 4px;
}
input[type="submit"] {
background-color: #4CAF50;
color: white;
padding: 10px 15px;
border: none;
border-radius: 4px;
cursor: pointer;
}
input[type="submit"]:hover {
background-color: #45a049;
}
</style>
</head>
<body>
<h2>Contact Us</h2>
<form action="/submit-form" method="post">
<fieldset>
<legend>Contact Information</legend>
<!-- Name field -->
<label for="name">Name:</label>
<input type="text" id="name" name="name" placeholder="Enter your full name" required>
<!-- Email field -->
<label for="email">Email:</label>
<input type="email" id="email" name="email" placeholder="Enter your email" required>
<!-- Message field -->
<label for="message">Message:</label>
<textarea id="message" name="message" rows="5" placeholder="Write your message here..." required></textarea>
<!-- Submit button -->
<input type="submit" value="Submit">
</fieldset>
</form>
</body>
</html>
Code Explanation:
- Basic HTML structure: The document starts with standard HTML tags (
<html>
,<head>
,<body>
). It includes basic styling in the<style>
section to improve the appearance of the form. - Form setup:
- The form is wrapped inside the
<form>
tag with theaction="/submit-form"
attribute, simulating a server-side submission. - The
method="post"
attribute is used, ensuring that the form data is sent in the request body.
- The form is wrapped inside the
- Input fields:
- The form includes three input fields:
name
(text),email
(email), andmessage
(textarea). - The
required
attribute is added to ensure that users cannot submit the form without completing all fields.
- The form includes three input fields:
- Label elements:
- The
<label>
tags are linked to their respective input fields using thefor
attribute, which matches theid
of each input. This enhances form accessibility.
- The
- Submit button:
- A submit button (
<input type="submit">
) is provided to allow users to submit the form.
- A submit button (
Project Summary:
By completing this project, you will gain hands-on experience in creating and structuring an HTML form, using various input types, and implementing form submission with proper action
and method
attributes. This form can serve as a foundation for more complex web forms you will build in future projects.
Interview Questions for Contact Form Project
Here are some interview questions based on the concepts and skills learned from building a contact form using HTML. These questions are designed to test the understanding of form structure, input types, form submission methods, and best practices in web development.
Basic Form Structure
- What is the purpose of the
<form>
element in HTML?- Answer: The
<form>
element in HTML defines a form for user input and acts as a container for form controls such as input fields, checkboxes, radio buttons, and submit buttons. It also specifies where the data should be sent and how it should be sent using attributes likeaction
andmethod
.
- Answer: The
- What are the commonly used attributes of the
<form>
element?- Answer: The two most commonly used attributes of the
<form>
element are:action
: Specifies the URL where the form data should be sent for processing.method
: Defines the HTTP method (GET
orPOST
) to be used when sending the form data.
- Answer: The two most commonly used attributes of the
Input Types
- Explain the difference between
type="text"
andtype="password"
in an<input>
element.- Answer: The
type="text"
input allows users to enter visible, single-line text, such as a username. In contrast,type="password"
masks the characters entered by the user (e.g., with asterisks or dots), making it suitable for sensitive information like passwords.
- Answer: The
- What input type would you use to create a field for email validation, and why?
- Answer: You would use
type="email"
for email validation. This input type requires the user to enter a properly formatted email address (e.g., containing “@” and a domain name) and performs basic validation to ensure the input follows the correct email structure.
- Answer: You would use
- How can you create a multi-line text input in HTML?
- Answer: To create a multi-line text input, you use the
<textarea>
element instead of an<input>
element. The<textarea>
allows users to enter longer, multi-line text such as a message or comment.
- Answer: To create a multi-line text input, you use the
Form Submission
- What is the difference between the
GET
andPOST
methods when submitting a form?- Answer:
- The
GET
method appends the form data to the URL as query parameters, making it visible and limited in size. It is best suited for retrieving data, such as in a search form. - The
POST
method sends the form data in the HTTP request body, making it more secure and suitable for sensitive or large amounts of data. It is commonly used for login, registration, and file upload forms.
- The
- Answer:
- When should you use the
POST
method for form submission?- Answer: The
POST
method should be used when submitting sensitive data (e.g., passwords or personal information), when the form data is large, or when you do not want the data to be visible in the URL. It is also appropriate when creating or modifying resources on the server.
- Answer: The
- What is the role of the
action
attribute in a form?- Answer: The
action
attribute specifies the URL or server endpoint where the form data will be sent for processing after the user submits the form. If theaction
attribute is not specified, the form data is sent to the same URL as the current page.
- Answer: The
Accessibility and Usability
- How do you associate a
<label>
with an<input>
element, and why is this important?- Answer: You associate a
<label>
with an<input>
by using thefor
attribute in the<label>
tag and matching it with theid
of the<input>
element. This association improves accessibility, as it allows users who rely on screen readers or other assistive technologies to understand what each input field is for. It also enhances the usability of forms, allowing users to click on the label to focus on the corresponding input field.
- Answer: You associate a
- What is the purpose of the
<fieldset>
and<legend>
elements in a form?- Answer: The
<fieldset>
element is used to group related form controls together, making the form more organized and easier to navigate. The<legend>
element provides a title or description for the group of form controls inside the<fieldset>
, helping users understand the purpose of that section. These elements are important for accessibility and improving the overall user experience.
- Answer: The
Best Practices
- Why is it important to use the
required
attribute in input fields, and what does it do?- Answer: The
required
attribute ensures that users must fill out a particular input field before submitting the form. This helps to prevent incomplete form submissions and ensures that essential data is collected. Without it, users may submit a form with missing information, leading to errors or incomplete data processing on the server.
- Answer: The
- Explain why it’s important to validate form data both on the client-side and server-side.
- Answer:
- Client-side validation helps improve user experience by providing instant feedback and preventing users from submitting invalid data (e.g., incorrectly formatted email addresses). It reduces the load on the server by catching errors early.
- Server-side validation is crucial for security and data integrity. Even if client-side validation is bypassed (e.g., through browser manipulation), server-side validation ensures that only valid and expected data is processed by the server, protecting against malicious inputs like SQL injection.
- Answer:
Practical Implementation
- Create an example of a contact form that collects a user’s name, email, and message. Explain the role of each element.
- Answer: (Candidate is expected to create a basic contact form like the one they built during the session and explain the purpose of each part, such as
<form>
,<input>
,<label>
, and how theaction
andmethod
attributes work.)
- Answer: (Candidate is expected to create a basic contact form like the one they built during the session and explain the purpose of each part, such as