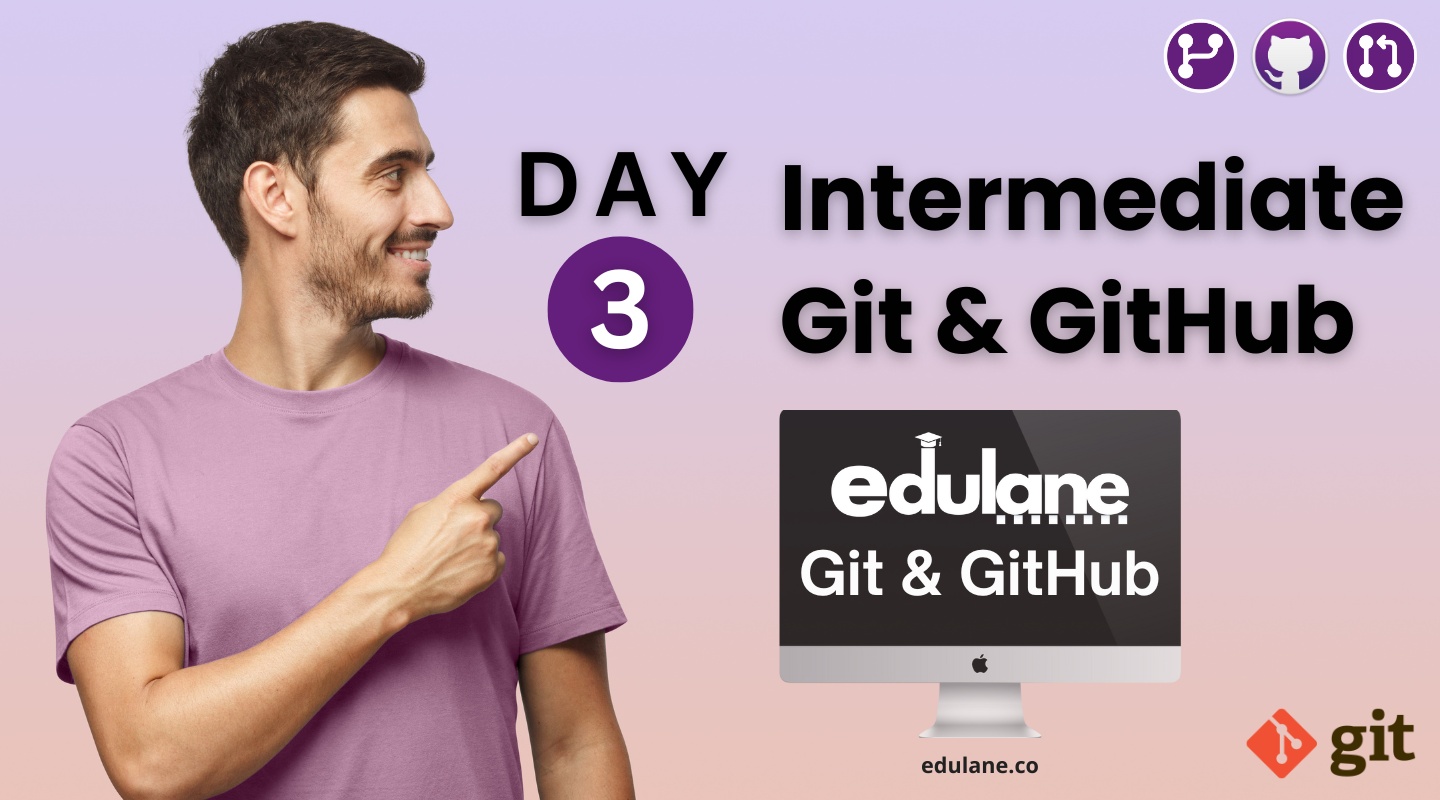
Mastering Git and GitHub: Advanced Techniques GitHub Actions & Workflows
Dive into the advanced features of Git and GitHub with this comprehensive workshop designed for developers who want to elevate their version control and project management skills. This workshop covers essential intermediate and advanced concepts, including:
- Branching and Merging: Learn how to create, manage, and merge branches to streamline feature development and bug fixes.
- Remote Repositories: Master adding, removing, fetching, pulling, and pushing changes with remote repositories.
- Working with GitHub: Explore forking, cloning, and creating pull requests to collaborate effectively on GitHub.
- Tagging: Understand how to create, list, and delete tags to manage releases and versions.
- Advanced GitHub Commands: Utilize GitHub CLI for efficient issue tracking and pull request management.
- Viewing Workflow Runs: Monitor and debug your GitHub Actions workflows with detailed logs and insights.
- Managing GitHub Actions: Create and configure automation workflows, manage secrets, and set environment variables.
- Snapshotting: Learn about Git snapshots to capture and view the state of your project at any point.
- Sharing & Updating Projects: Share your work with others and keep your local repository up-to-date with remote changes.
- Inspection & Comparison: Inspect changes and compare different commits and branches to maintain code quality.
- Git Stash: Use
git stash
to temporarily save and manage uncommitted changes without disrupting your workflow.
Table of Contents
- GitHub Advanced Techniques
- GitHub Workflow and Actions
- Snapshotting
- Sharing & Updating Projects
- Inspection & Comparison
- Git Stash
GitHub Advanced Techniques
This section covers advanced GitHub techniques to enhance your workflow, collaboration, and project management capabilities. These techniques include managing branches and merges, working with remote repositories, tagging, viewing workflow runs, managing GitHub Actions, and utilizing snapshotting, sharing, and updating projects.
Branching and Merging
Creating Branches
To start working on a new feature for the Edulane project, create a new branch:
git branch feature/new-dashboard
To create and switch to the branch in one command:
git checkout -b feature/new-dashboard
Listing Branches
To view all local branches:
git branch
To view remote branches:
git branch -r
Switching Branches
To switch to the feature/new-dashboard
branch:
git checkout feature/new-dashboard
Merging Branches
To merge feature/new-dashboard
into main
:
- Switch to
main
:
git checkout main
- Merge the feature branch:
git merge feature/new-dashboard
- Resolve any conflicts if prompted.
Rebasing
To rebase feature/new-dashboard
onto main
:
- Switch to
feature/new-dashboard
:
git checkout feature/new-dashboard
- Rebase onto
main
:
git rebase main
- Switch to
main
and fast-forward:
git checkout main git merge feature/new-dashboard
Remote Repositories
Adding and Removing Remotes
To add a new remote:
git remote add origin https://github.com/edulane/repo.git
To remove a remote:
git remote remove origin
Fetching and Pulling
To fetch changes from the origin
remote:
git fetch origin
To pull changes from main
:
git pull origin main
Pushing Changes
To push changes to the main
branch:
git push origin main
Working with GitHub
Forking and Cloning
To clone your forked repository:
git clone https://github.com/your-username/edulane.git
Creating a Pull Request
- Push your branch to GitHub:
git push origin feature/new-dashboard
- Go to the GitHub repository and create a pull request by selecting your branch and providing a description.
Tagging
What is Tagging in GitHub?
Tagging in GitHub is a way to mark specific points in your repository’s history as important. Tags are often used to mark release versions (like v1.0.0
), but they can also be used for any other significant points in your project’s development. Tags are immutable, meaning once they are created, they do not change, providing a reliable reference point in the project history.
Types of Tags
- Lightweight Tags:
- Simply a name that points to a specific commit.
- Created quickly and with minimal metadata.
- Annotated Tags:
- Contains a full object that includes the tagger’s name, email, date, and a tagging message.
- Can be cryptographically signed and verified.
Creating Tags
Creating a Lightweight Tag
You can create a lightweight tag using the following command:
git tag <tagname>
Example:
git tag v1.0
Creating an Annotated Tag
Annotated tags are recommended because they store extra metadata about the tag:
git tag -a <tagname> -m "Tag message"
Example:
git tag -a v1.0 -m "Version 1.0 release"
Pushing Tags to Remote
After creating a tag, you need to push it to your remote repository to make it available to others.
Pushing a Specific Tag
git push origin <tagname>
Example:
git push origin v1.0.0
Pushing All Tags
git push --tags
Checking Out Tags
To check out a specific tag, which puts you in a detached HEAD state:
git checkout <tagname>
Example:
git checkout v1.0.0
Listing and Deleting Tags
To list tags:
git tag
To delete a tag:
git tag -d v1.0
GitHub Workflow and Actions
What is GitHub Workflow?
A GitHub Workflow is a configurable automated process made possible by GitHub Actions that can run on events in your GitHub repository. Workflows are defined using YAML syntax and stored in the .github/workflows
directory of your repository. They can perform a wide variety of tasks, such as building and testing your code, deploying your applications, or automating other project management tasks.
Key Features of GitHub Workflows
- Event-Driven: Workflows are triggered by events, which can be anything from pushing code, creating pull requests, issues, or even on a schedule.
- Automation: Automate repetitive tasks, such as running tests, building code, and deploying applications.
- Customizable: Define custom workflows tailored to your project needs using a combination of pre-defined and custom actions.
- Concurrent Jobs: Run multiple jobs in parallel to speed up the execution of your workflows.
- Environment Management: Manage different environments, secrets, and configurations to ensure your workflows run correctly across various stages of your project.
Components of a GitHub Workflow
- Events: These are the triggers that start a workflow. Common events include
push
,pull_request
,schedule
, andworkflow_dispatch
. - Jobs: A workflow can have multiple jobs, which run in parallel by default. Jobs consist of steps that are executed in sequence.
- Steps: Each job is made up of steps, which are individual tasks like running a script, setting up a dependency, or executing a shell command. Steps can use actions, which are reusable units of code defined by the community or custom actions created by you.
- Actions: Actions are standalone commands that are combined into steps to create a job. GitHub provides a marketplace with many pre-built actions.
Example Workflow
Here’s an example workflow for a Node.js project that runs tests on every push to the main
branch.
name: CI
on:
push:
branches:
- main
pull_request:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout repository
uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
Detailed Breakdown
- Name:
- The name of the workflow (
CI
in this case).
- The name of the workflow (
- on:
- Specifies the events that trigger the workflow. Here, it triggers on
push
andpull_request
events to themain
branch.
- Specifies the events that trigger the workflow. Here, it triggers on
- jobs:
- Defines the jobs to run. In this example, there is one job called
build
.
- Defines the jobs to run. In this example, there is one job called
- runs-on:
- Specifies the type of machine to run the job on.
ubuntu-latest
is a common choice for Linux-based workflows.
- Specifies the type of machine to run the job on.
- steps:
- Contains a list of steps to execute. Each step can use an action or run a command:
- Checkout: Uses the
actions/checkout
action to clone the repository. - Set up Node.js: Uses the
actions/setup-node
action to set up the Node.js environment. - Install dependencies: Runs
npm install
to install project dependencies. - Run tests: Runs
npm test
to execute the project’s tests.
- Checkout: Uses the
- Contains a list of steps to execute. Each step can use an action or run a command:
Advanced Concepts
- Matrix Builds:
- Run jobs with different configurations in parallel, like testing multiple versions of Node.js
strategy:
matrix:
node-version: [10, 12, 14]
- Conditional Execution:
- Use the
if
key to run steps based on conditions.
- Use the
if: github.event_name == 'push'
- Secrets and Environment Variables:
- Manage sensitive information and environment-specific configurations.
env:
NODE_ENV: production
- name: Deploy
run: ./deploy.sh
env:
SECRET_KEY: ${{ secrets.DEPLOYMENT_KEY }}
GitHub Workflows are a powerful tool to automate and streamline your development process. By defining workflows tailored to your project’s needs, you can ensure consistency, efficiency, and reliability in your CI/CD pipeline. If you have more specific scenarios or need further examples, feel free to ask!
- Reusable Workflows:
- Create workflows that can be reused across multiple repositories or projects.
What is GitHub Actions?
GitHub Actions is a powerful, flexible CI/CD (Continuous Integration and Continuous Deployment) platform integrated directly into GitHub. It allows you to automate your workflows, such as building, testing, and deploying your code, right from your GitHub repository. GitHub Actions supports event-driven automation, meaning it can run a series of commands in response to events that happen within your GitHub repository.
Key Features of GitHub Actions
- Automation of Workflows: Automate repetitive tasks and workflows, such as code testing, deployment, and more.
- Customizable and Scalable: Define custom workflows using YAML syntax, tailored to your specific needs.
- Integration with GitHub Ecosystem: Seamlessly integrates with other GitHub features like pull requests, issues, and releases.
- Reusable Components: Leverage a wide array of community-contributed actions available in the GitHub Marketplace.
- Concurrent and Parallel Execution: Run multiple jobs in parallel, reducing the time required for CI/CD pipelines.
- Cross-Platform Support: Execute workflows on different operating systems like Linux, macOS, and Windows.
Components of GitHub Actions
- Workflows:
- A workflow is a configurable automated process that you set up in your repository. Workflows are defined using YAML files stored in the
.github/workflows
directory.
- A workflow is a configurable automated process that you set up in your repository. Workflows are defined using YAML files stored in the
- Events:
- Events are specific activities that trigger workflows, such as
push
,pull_request
, orschedule
(for cron jobs).
- Events are specific activities that trigger workflows, such as
- Jobs:
- A job is a set of steps that execute on the same runner. Jobs run in parallel by default, but you can configure them to run sequentially.
- Steps:
- Steps are individual tasks within a job. Each step can run commands or actions.
- Actions:
- Actions are standalone commands that can be combined into steps. You can use actions from the GitHub Marketplace or create custom actions.
Example of GitHub Actions Workflow
Here’s an example of a simple GitHub Actions workflow for a Node.js project that runs tests on every push to the main
branch.
name: CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout repository
uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
Detailed Breakdown
- name:
- The name of the workflow (
CI
in this case).
- The name of the workflow (
- on:
- Specifies the events that trigger the workflow. This workflow is triggered by
push
andpull_request
events to themain
branch.
- Specifies the events that trigger the workflow. This workflow is triggered by
- jobs:
- Defines the jobs to run. This workflow has a single job named
build
.
- Defines the jobs to run. This workflow has a single job named
- runs-on:
- Specifies the type of machine to run the job on. Here, it’s
ubuntu-latest
.
- Specifies the type of machine to run the job on. Here, it’s
- steps:
- Contains a list of steps to execute. Each step can use actions or run commands:
- Checkout repository: Uses the
actions/checkout
action to clone the repository. - Set up Node.js: Uses the
actions/setup-node
action to set up Node.js. - Install dependencies: Runs
npm install
to install dependencies. - Run tests: Runs
npm test
to execute tests.
- Checkout repository: Uses the
- Contains a list of steps to execute. Each step can use actions or run commands:
Advanced Features
Matrix Builds
Matrix builds allow you to run jobs with different configurations in parallel, such as testing against multiple versions of Node.js.
strategy:
matrix:
node-version: [10, 12, 14]
Conditional Execution
Use the if
key to conditionally run steps based on certain conditions.
steps:
- name: Run tests
run: npm test
if: github.event_name == 'push'
Secrets and Environment Variables
Manage sensitive information and configuration settings using secrets and environment variables.
env:
NODE_ENV: production
- name: Deploy
run: ./deploy.sh
env:
SECRET_KEY: ${{ secrets.DEPLOYMENT_KEY }}
Reusable Workflows
Create workflows that can be reused across multiple repositories or projects, making your CI/CD pipelines more efficient and consistent.
Using GitHub Actions in Real Projects
Here’s how you can use GitHub Actions to improve your development workflow for an Edulane project:
- Automate Testing:
- Automatically run your test suite on every push or pull request to ensure code quality.
- Continuous Deployment:
- Deploy your application to production or staging environments automatically when changes are merged into the
main
branch.
- Deploy your application to production or staging environments automatically when changes are merged into the
- Code Quality Checks:
- Integrate tools like ESLint or Prettier to enforce code style and quality standards.
- Notification Systems:
- Set up notifications to inform your team about build statuses or deployment results through Slack, email, or other communication tools.
Example: Automated Deployment Workflow
Here’s an example workflow that not only runs tests but also deploys to a production environment if the tests pass:
name: CI and Deploy
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout repository
uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
- name: Deploy to production
if: success()
run: ./deploy.sh
env:
SECRET_KEY: ${{ secrets.DEPLOYMENT_KEY }}
In this example:
- The
Deploy to production
step runs only if all previous steps succeed (if: success()
). - It uses a secret for deployment keys, ensuring sensitive information is kept secure.
GitHub Actions provide a robust and flexible way to automate and enhance your development workflows, making them an essential tool for modern software development. Whether you’re looking to automate tests, deployments, or other project management tasks, GitHub Actions can help you achieve a more efficient and reliable workflow. If you have any more questions or need specific examples, feel free to ask!
Viewing Workflow Runs
Accessing Workflow Runs
- Go to your GitHub repository.
- Click on the “Actions” tab.
Reviewing Logs
- Click on a specific workflow run to see its details.
- Click on jobs within the run to view logs.
- Use search and filter features to find specific outputs or errors.
Managing GitHub Actions
Creating and Configuring Workflows
Create a workflow file in .github/workflows/
named ci.yml
:
name: CI
on:
push:
branches:
- main
pull_request:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
Managing Secrets and Environment Variables
Adding Secrets
- Go to GitHub repository settings.
- Click on “Secrets” under the “Security” section.
- Add new repository secrets like API keys.
Using Secrets in Workflows
Reference secrets in workflow files:
- name: Deploy
run: ./deploy.sh
env:
SECRET_KEY: ${{ secrets.DEPLOYMENT_KEY }}
Configuring Environment Variables
Set environment variables in your workflow:
jobs:
build:
env:
NODE_ENV: production
Snapshotting
Snapshotting in Git refers to how Git records the state of your files at a particular point in time. Each commit you make in Git is essentially a snapshot of your repository at that moment.
Creating Snapshots
When you commit changes, Git creates a snapshot of your files:
git add .
git commit -m "Snapshot of project before major update"
This snapshot is saved in your commit history and can be viewed or reverted to at any time.
Viewing Snapshots
To view the history of snapshots:
git log
You can see the details of each snapshot by using:
git show <commit-hash>
Sharing & Updating Projects
Sharing Your Work
- Push Changes to Remote
- Collaborate on Remote Branches
Create a new branch on the remote and push changes:
git push origin feature/new-feature
Updating Your Local Repository
To update your local repository with changes from the remote:
- Fetch Changes
git fetch origin
- Merge or RebaseMerge changes:
git merge origin/main
Or rebase:
git rebase origin/main
Inspection & Comparison
Viewing Changes
To see changes between your working directory and the last commit:
git status
To view changes in detail:
git diff
Comparing Commits
To compare two commits:
git diff <commit1> <commit2>
Comparing Branches
To compare changes between branches:
git diff branch1..branch2
Git Stash
What is Git Stash?
Git stash is a feature that allows you to temporarily save changes that you are not ready to commit. This is useful when you need to switch branches or work on something else, but you want to come back to your current work later without committing it.
Key Features of Git Stash
- Temporary Storage: Temporarily store changes in your working directory that are not yet ready for a commit.
- Non-Destructive: Changes are stashed and can be reapplied later, preserving your work-in-progress.
- Multiple Stashes: You can have multiple stashes, and each one can be managed separately.
Common Git Stash Commands
Stashing Changes
If you need to temporarily set aside changes you’ve made, you can use git stash
:
git stash
This command saves your uncommitted changes and reverts your working directory to match the HEAD commit.
Stashing with a Message
You can add a message to identify the stash:
git stash save "message describing the changes"
Listing Stashes
To list all stashed changes:
git stash list
Applying a Stash
To apply the most recent stash:
git stash apply
To apply a specific stash:
git stash apply stash@{index}
Dropping a Stash
To remove a specific stash:
git stash drop stash@{index}
To remove all stashes:
git stash clear
Popping a Stash
To apply and remove the most recent stash:
git stash pop