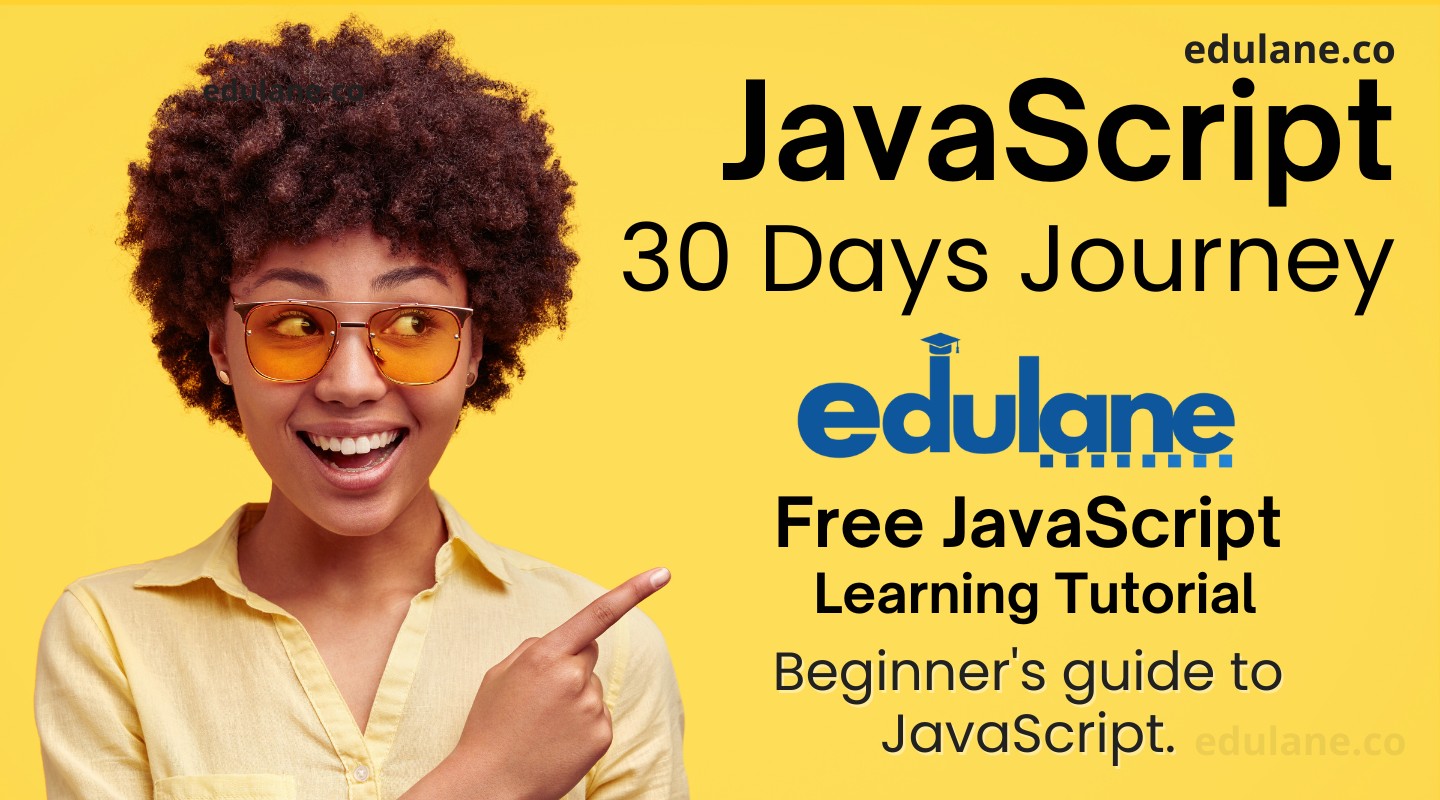
Free JavaScript Learning Tutorial
Welcome to the 30-Days JavaScript Learning Journey! This plan will take you from the basics of JavaScript to more advanced concepts, with practical examples and mini-projects along the way.
Table of contents
- Day 1-5: Basics of JavaScript
- Day 6-10: Functions and Arrays
- Day 11-15: Intermediate Concepts
- Day 16-20: Advanced Topics
- Day 21-25: Projects
- Day 26-30: Final Touches and Deployment
- How to Use This Guide
Day 1-5: Basics of JavaScript
Day 1: Introduction to JavaScript
- What is JavaScript?
- Setting up the development environment (Node.js, VS Code)
- Writing your first JavaScript program (Hello, World!)
- Basic syntax and comments
- Example: Displaying “Hello, World!” in the console
Day 2: Variables and Data Types
- Variables:
var
,let
, andconst
- Data types: Number, String, Boolean, Null, Undefined, Object
- Example: Creating variables of different types and logging them
Day 3: Operators
- Arithmetic operators
- Comparison operators
- Logical operators
- Assignment operators
- Example: Simple calculator using arithmetic operators
Day 4: Conditional Statements
if
,else if
,else
switch
statement- Example: Program to check if a number is positive, negative, or zero
Day 5: Loops
for
loopwhile
loopdo...while
loop- Example: Printing numbers from 1 to 10 using different loops

Day 6-10: Functions and Arrays
Day 6: Functions
- Declaring and invoking functions
- Parameters and return values
- Arrow functions
- Example: Function to calculate the sum of two numbers
Day 7: Scope and Hoisting
- Local and global scope
- Hoisting
- Example: Demonstrating scope with nested functions
Day 8: Arrays
- Creating and accessing arrays
- Array methods:
push
,pop
,shift
,unshift
,length
- Example: Managing a list of favorite movies
Day 9: Array Iteration
for
loop for arraysforEach
methodmap
,filter
,reduce
- Example: Filtering an array of numbers to get only even numbers
Day 10: Objects
- Creating and accessing objects
- Object methods
this
keyword- Example: Creating an object to store user information
Day 11-15: Intermediate Concepts
Day 11: Strings
- String properties and methods
- Template literals
- Example: Manipulating and formatting strings
Day 12: Date and Time
- Date object
- Methods for getting and setting date values
- Example: Displaying the current date and time
Day 13: Math Object
- Common Math methods
- Example: Creating a random number generator
Day 14: Events
- Event listeners (
addEventListener
) - Event object
- Example: Handling button click events to change text on the page
Day 15: DOM Manipulation
- Selecting elements (
getElementById
,querySelector
) - Modifying elements (
innerHTML
,style
) - Example: Changing the content and style of a web page element
Day 16-20: Advanced Topics
Day 16: JSON
- JSON format
- Parsing JSON
- Stringifying JSON
- Example: Working with JSON data
Day 17: Error Handling
try...catch
statement- Throwing errors
- Example: Error handling in a simple calculator
Day 18: Asynchronous JavaScript
- Callbacks
- Promises
async
andawait
- Example: Fetching data from an API using Promises and
async/await
Day 19: Fetch API
- Using the Fetch API
- Handling responses and errors
- Example: Making a GET request to an API
Day 20: Local Storage
- Storing data in local storage
- Retrieving data from local storage
- Example: Storing and retrieving user preferences

Day 21-25: Projects
Day 21: To-Do List App (Part 1)
- Setting up the project
- HTML structure and basic CSS
- Adding tasks
Day 22: To-Do List App (Part 2)
- Marking tasks as complete
- Deleting tasks
- Example: Building a functional to-do list app
Day 23: Weather App (Part 1)
- Setting up the project
- Fetching weather data from an API
- Displaying weather information
Day 24: Weather App (Part 2)
- Handling user input (city name)
- Updating the UI with fetched data
- Example: Building a weather app that shows current weather for a city
Day 25: Quiz App (Part 1)
- Setting up the project
- HTML structure and basic CSS
- Creating and displaying questions
Day 26-30: Final Touches and Deployment
Day 26: Quiz App (Part 2)
- Handling user answers
- Calculating and displaying scores
- Example: Building a functional quiz app
Day 27: Debugging
- Debugging techniques
- Using browser developer tools
- Example: Debugging common issues in a web app
Day 28: Performance Optimization
- Tips for optimizing JavaScript performance
- Example: Improving the performance of the to-do list app
Day 29: ES6+ Features
- New features in ES6 and beyond (e.g., destructuring, modules, classes)
- Example: Refactoring code to use modern JavaScript features
Day 30: Deployment
- Hosting a website on GitHub Pages
- Example: Deploying the to-do list app
How to Use This Guide
- Follow the day-by-day plan to learn JavaScript.
- Implement the examples and mini-projects to practice what you’ve learned.
- Adjust the pace based on your learning needs and prior knowledge.
Happy Learning!