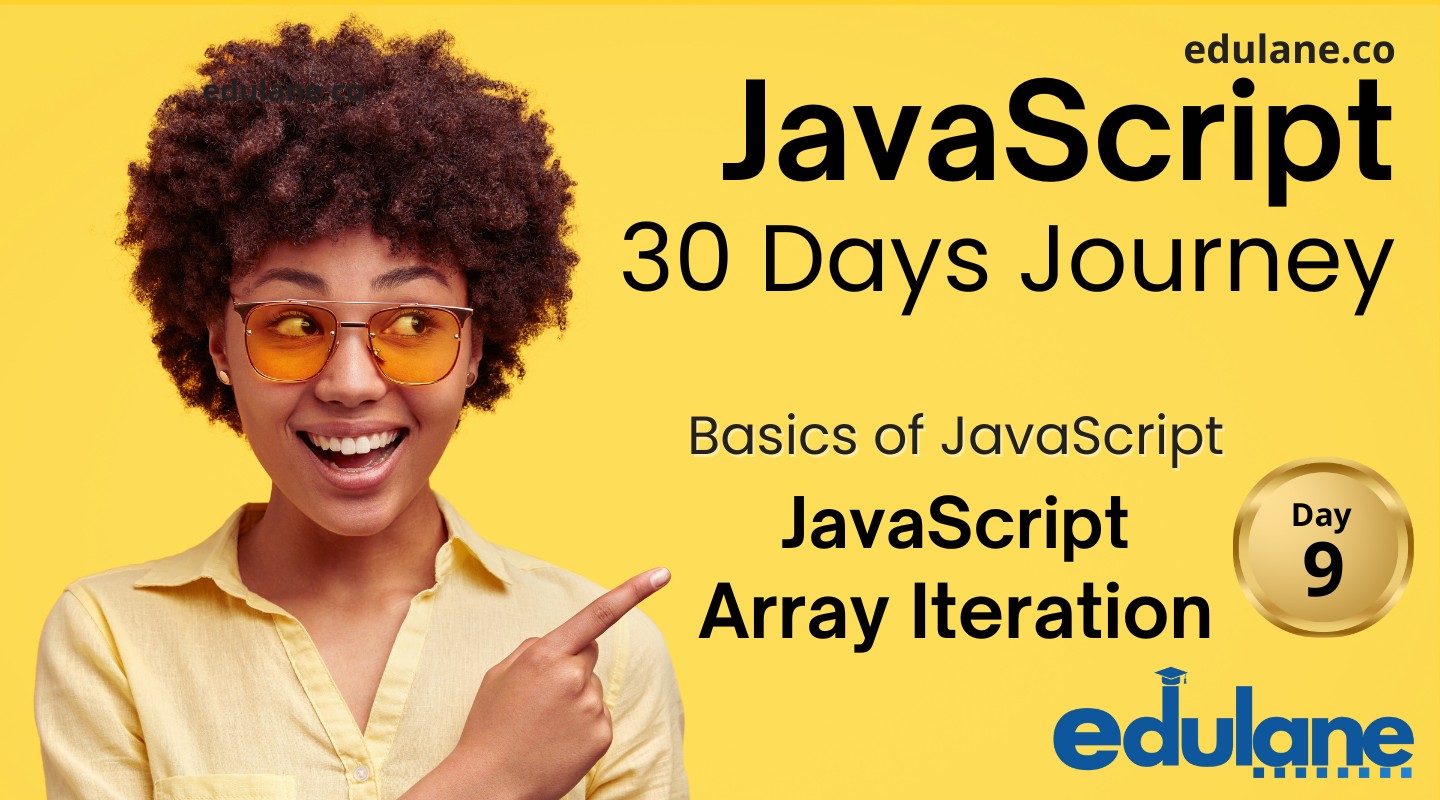
Table of contents
- Introduction to Array Iteration
- Basic Iteration Methods
for
loopwhile
loopdo...while
loop
- ES5 Iteration Methods
- forEach()
- ES6+ Iteration Methods
map()
filter()
reduce()
some()
every()
find()
findIndex(
)
- Advanced Iteration Techniques
- Nested iteration
- Combining iteration methods
- Using
reduce()
for complex transformations
- Performance Considerations
- When to use which method
- Performance comparisons
- Common Pitfalls and How to Avoid Them
- Avoiding mutations
- Dealing with asynchronous operations in iterations
- Best Practices
- Immutable operations
- Clarity and readability
- Avoiding side effects
Detailed Guide
1. Introduction to Array Iteration
Array iteration refers to the process of traversing or looping through the elements of an array to perform operations such as accessing, modifying, or evaluating each element.
Basic Iteration Methods
for
loop:
const arr = [1, 2, 3, 4, 5];
// Example 1: Simple iteration
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
// Output: 1 2 3 4 5
// Example 2: Calculating sum of elements
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
console.log(sum);
// Output: 15
// Example 3: Creating a new array with doubled values
const doubled = [];
for (let i = 0; i < arr.length; i++) {
doubled.push(arr[i] * 2);
}
console.log(doubled);
// Output: [2, 4, 6, 8, 10]
while
loop:
const arr = [1, 2, 3, 4, 5];
// Example 1: Simple iteration
let i = 0;
while (i < arr.length) {
console.log(arr[i]);
i++;
}
// Output: 1 2 3 4 5
// Example 2: Calculating product of elements
let product = 1;
i = 0;
while (i < arr.length) {
product *= arr[i];
i++;
}
console.log(product);
// Output: 120
// Example 3: Filtering even numbers
const evens = [];
i = 0;
while (i < arr.length) {
if (arr[i] % 2 === 0) {
evens.push(arr[i]);
}
i++;
}
console.log(evens);
// Output: [2, 4]
do...while
loop:
const arr = [1, 2, 3, 4, 5];
// Example 1: Simple iteration
let i = 0;
do {
console.log(arr[i]);
i++;
} while (i < arr.length);
// Output: 1 2 3 4 5
// Example 2: Finding first element greater than 3
i = 0;
let found;
do {
if (arr[i] > 3) {
found = arr[i];
break;
}
i++;
} while (i < arr.length);
console.log(found);
// Output: 4
// Example 3: Creating a new array with squared values
const squared = [];
i = 0;
do {
squared.push(arr[i] * arr[i]);
i++;
} while (i < arr.length);
console.log(squared);
// Output: [1, 4, 9, 16, 25]
3. ES5 Iteration Methods
forEach()
:
const arr = [1, 2, 3, 4, 5];
// Example 1: Simple iteration
arr.forEach(element => console.log(element));
// Output: 1 2 3 4 5
// Example 2: Calculating sum of elements
let sum = 0;
arr.forEach(element => sum += element);
console.log(sum);
// Output: 15
// Example 3: Modifying elements in place
arr.forEach((element, index, array) => array[index] = element * 2);
console.log(arr);
// Output: [2, 4, 6, 8, 10]
4. ES6+ Iteration Methods
map()
:
const arr = [1, 2, 3, 4, 5];
// Example 1: Doubling values
const doubled = arr.map(element => element * 2);
console.log(doubled);
// Output: [2, 4, 6, 8, 10]
// Example 2: Converting numbers to strings
const strings = arr.map(element => element.toString());
console.log(strings);
// Output: ['1', '2', '3', '4', '5']
// Example 3: Extracting property values from objects
const objects = [{ id: 1 }, { id: 2 }, { id: 3 }];
const ids = objects.map(obj => obj.id);
console.log(ids);
// Output: [1, 2, 3]
filter()
:
const arr = [1, 2, 3, 4, 5];
// Example 1: Filtering even numbers
const evens = arr.filter(element => element % 2 === 0);
console.log(evens);
// Output: [2, 4]
// Example 2: Filtering odd numbers
const odds = arr.filter(element => element % 2 !== 0);
console.log(odds);
// Output: [1, 3, 5]
// Example 3: Filtering numbers greater than 2
const greaterThanTwo = arr.filter(element => element > 2);
console.log(greaterThanTwo);
// Output: [3, 4, 5]
reduce()
:
const arr = [1, 2, 3, 4, 5];
// Example 1: Calculating sum of elements
const sum = arr.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum);
// Output: 15
// Example 2: Calculating product of elements
const product = arr.reduce((accumulator, currentValue) => accumulator * currentValue, 1);
console.log(product);
// Output: 120
// Example 3: Finding maximum element
const max = arr.reduce((max, currentValue) => currentValue > max ? currentValue : max, arr[0]);
console.log(max);
// Output: 5
some()
:
const arr = [1, 2, 3, 4, 5];
// Example 1: Checking if any element is even
const hasEven = arr.some(element => element % 2 === 0);
console.log(hasEven);
// Output: true
// Example 2: Checking if any element is greater than 5
const hasGreaterThanFive = arr.some(element => element > 5);
console.log(hasGreaterThanFive);
// Output: false
// Example 3: Checking if any element is a negative number
const hasNegative = arr.some(element => element < 0);
console.log(hasNegative);
// Output: false
every()
:
const arr = [1, 2, 3, 4, 5];
// Example 1: Checking if all elements are positive
const allPositive = arr.every(element => element > 0);
console.log(allPositive);
// Output: true
// Example 2: Checking if all elements are even
const allEven = arr.every(element => element % 2 === 0);
console.log(allEven);
// Output: false
// Example 3: Checking if all elements are less than 10
const allLessThanTen = arr.every(element => element < 10);
console.log(allLessThanTen);
// Output: true
find()
:
const arr = [1, 2, 3, 4, 5];
// Example 1: Finding first element greater than 3
const found = arr.find(element => element > 3);
console.log(found);
// Output: 4
// Example 2: Finding first even element
const firstEven = arr.find(element => element % 2 === 0);
console.log(firstEven);
// Output: 2
// Example 3: Finding first element less than 0
const negative = arr.find(element => element < 0);
console.log(negative);
// Output: undefined
findIndex()
const arr = [1, 2, 3, 4, 5];
// Example 1: Finding index of first element greater than 3
const index = arr.findIndex(element => element > 3);
console.log(index);
// Output: 3
// Example 2: Finding index of first even element
const evenIndex = arr.findIndex(element => element % 2 === 0);
console.log(evenIndex);
// Output: 1
// Example 3: Finding index of first element less than 0
const negativeIndex = arr.findIndex(element => element < 0);
console.log(negativeIndex);
// Output: -1
5. Advanced Iteration Techniques
Nested iteration:
const matrix = [
[1, 2],
[3, 4],
[5, 6]
];
// Example 1: Iterating over a 2D array
matrix.forEach(row => {
row.forEach(cell => {
console.log(cell);
});
});
// Output: 1 2 3 4 5 6
// Example 2: Calculating the sum of all elements in a 2D array
let sum = 0;
matrix.forEach(row => {
row.forEach(cell => {
sum += cell;
});
});
console.log(sum);
// Output: 21
// Example 3: Flattening a 2D array
const flattened = [];
matrix.forEach(row => {
row.forEach(cell => {
flattened.push(cell);
});
});
console.log(flattened);
// Output: [1, 2, 3, 4, 5, 6]
Combining iteration methods:
const arr = [1, 2, 3, 4, 5];
// Example 1: Filtering odd numbers and doubling them
const result = arr
.filter(element => element % 2 !== 0)
.map(element => element * 2);
console.log(result);
// Output: [2, 6, 10]
// Example 2: Filtering even numbers and calculating their sum
const sumOfEvens = arr
.filter(element => element % 2 === 0)
.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sumOfEvens);
// Output: 6
// Example 3: Finding if any doubled odd number is greater than 6
const isAnyDoubledOddGreaterThanSix = arr
.filter(element => element % 2 !== 0)
.map(element => element * 2)
.some(element => element > 6);
console.log(isAnyDoubledOddGreaterThanSix);
// Output: true
Using reduce()
for complex transformations:
const arr = [1, 2, 3, 4, 5];
// Example 1: Grouping elements by parity (odd/even)
const groupedByParity = arr.reduce((acc, currentValue) => {
const key = currentValue % 2 === 0 ? 'even' : 'odd';
if (!acc[key]) acc[key] = [];
acc[key].push(currentValue);
return acc;
}, {});
console.log(groupedByParity);
// Output: { odd: [1, 3, 5], even: [2, 4] }
// Example 2: Counting occurrences of each element
const arr2 = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4];
const counts = arr2.reduce((acc, currentValue) => {
acc[currentValue] = (acc[currentValue] || 0) + 1;
return acc;
}, {});
console.log(counts);
// Output: { 1: 1, 2: 2, 3: 3, 4: 4 }
// Example 3: Creating an object with keys as array values and values as their indices
const arr3 = ['a', 'b', 'c'];
const obj = arr3.reduce((acc, currentValue, index) => {
acc[currentValue] = index;
return acc;
}, {});
console.log(obj);
// Output: { a: 0, b: 1, c: 2 }
6. Performance Considerations
When to use which method:
- Use
for
loops for maximum control and performance. - Use higher-order functions like
map()
,filter()
, andreduce()
for readability and functional programming style.
Performance comparisons:
const arr = Array(1000000).fill(1);
// Example 1: Using `for` loop
console.time('for loop');
let sumFor = 0;
for (let i = 0; i < arr.length; i++) {
sumFor += arr[i];
}
console.timeEnd('for loop'); // Output: for loop: <time>ms
// Example 2: Using `forEach`
console.time('forEach');
let sumForEach = 0;
arr.forEach(element => sumForEach += element);
console.timeEnd('forEach'); // Output: forEach: <time>ms
// Example 3: Using `reduce`
console.time('reduce');
const sumReduce = arr.reduce((acc, currentValue) => acc + currentValue, 0);
console.timeEnd('reduce'); // Output: reduce: <time>ms
7. Common Pitfalls and How to Avoid Them
Avoiding mutations:
const arr = [1, 2, 3, 4, 5];
// Example 1: Using `map` for transformations instead of mutating
const doubled = arr.map(element => element * 2);
console.log(arr); // Output: [1, 2, 3, 4, 5]
console.log(doubled); // Output: [2, 4, 6, 8, 10]
Dealing with asynchronous operations in iterations:
const arr = [1, 2, 3, 4, 5];
// Example 1: Using `forEach` with async/await
async function asyncFunction(element) {
return element * 2;
}
async function processArray() {
for (const element of arr) {
const result = await asyncFunction(element);
console.log(result);
}
}
processArray();
// Output: 2 4 6 8 10
8. Best Practices
Immutable operations:
- Prefer methods like
map()
,filter()
, andreduce()
for transformations to avoid mutating the original array.
Clarity and readability:
- Use descriptive variable names and avoid deeply nested iterations for better readability.
Avoiding side effects:
- Keep functions pure and avoid modifying external state within iteration methods.
By following these guidelines and understanding the different methods and their use cases, you’ll be able to effectively and efficiently iterate over arrays in JavaScript.
Interview Questions:-
Here are 20 interview questions and answers based on JavaScript operators:
1. What is the purpose of the forEach
method in JavaScript arrays?
Answer: The forEach
method executes a provided function once for each array element. It does not return a new array.
2. How do you use the map
method on an array?
Answer: The map
method creates a new array populated with the results of calling a provided function on every element in the calling array. Example:
const numbers = [1, 2, 3];
const doubled = numbers.map(num => num * 2);
console.log(doubled); // [2, 4, 6]
3. What is the difference between map
and forEach
?
Answer: map
returns a new array with the results of applying a function to each element, whereas forEach
simply executes a function for each element without returning a new array.
4. How can you iterate over an array using a for...of
loop?
Answer: The for...of
loop iterates over the values of an iterable object, such as an array. Example:
const array = [10, 20, 30];
for (const value of array) {
console.log(value);
}
5. What does the reduce
method do?
Answer: The reduce
method executes a reducer function (that you provide) on each element of the array, resulting in a single output value. Example:
const sum = [1, 2, 3, 4].reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum); // 10
6. What is the difference between some
and every
methods?
Answer: The some
method checks if at least one element in the array passes the test implemented by the provided function and returns a boolean. The every
method checks if all elements pass the test and also returns a boolean.
7. How can you use the find
method on an array?
Answer: The find
method returns the value of the first element in the array that satisfies the provided testing function. If no elements satisfy the testing function, undefined
is returned.
8. Describe how the findIndex
method works.
Answer: The findIndex
method returns the index of the first element in the array that satisfies the provided testing function. If no elements satisfy the testing function, -1
is returned.
9. What is the purpose of the includes
method in arrays?
Answer: The includes
method determines whether an array includes a certain value among its entries, returning true
or false
as appropriate.
10. How do you use the indexOf
method?
Answer: The indexOf
method returns the first index at which a given element can be found in the array, or -1
if it is not present.
11. What is the entries
method and how is it used?
Answer: The entries
method returns a new Array Iterator object that contains the key/value pairs for each index in the array. It can be used with for...of
to iterate over both keys and values.
const array = ['a', 'b', 'c'];
for (const [index, value] of array.entries()) {
console.log(index, value);
}
12. How do you use the keys
method on an array?
Answer: The keys
method returns a new Array Iterator object that contains the keys for each index in the array.
const array = ['a', 'b', 'c'];
for (const key of array.keys()) {
console.log(key);
}
13. Explain the values
method.
Answer: The values
method returns a new Array Iterator object that contains the values for each index in the array.
const array = ['a', 'b', 'c'];
for (const value of array.values()) {
console.log(value);
}
14. How can you use Array.from
to create an array from an iterable object?
Answer: Array.from
creates a new, shallow-copied array instance from an array-like or iterable object.
const set = new Set(['a', 'b', 'c']);
const array = Array.from(set);
console.log(array); // ['a', 'b', 'c']
15. What are the differences between map
, forEach
, filter
, and reduce
?
Answer: map
returns a new array with the results of applying a function to each element. forEach
performs a function on each element but does not return a new array. filter
returns a new array with elements that pass a test implemented by a function. reduce
accumulates array elements into a single value by applying a function.
16. How can you use array iteration methods to flatten a nested array?
Answer: You can use reduce
in combination with concat
to flatten a nested array.
const nestedArray = [[1, 2], [3, 4], [5, 6]];
const flatArray = nestedArray.reduce((acc, val) => acc.concat(val), []);
console.log(flatArray); // [1, 2, 3, 4, 5, 6]
17. How do you find the maximum value in an array using reduce
?
Answer: You can use reduce
to iterate through the array and compare values to find the maximum.
const array = [1, 2, 3, 4, 5];
const max = array.reduce((a, b) => Math.max(a, b));
console.log(max); // 5
18. Can you explain how to remove duplicates from an array using iteration methods?
Answer: You can use filter
along with indexOf
to remove duplicates.
const array = [1, 2, 2, 3, 4, 4, 5];
const uniqueArray = array.filter((value, index, self) => self.indexOf(value) === index);
console.log(uniqueArray); // [1, 2, 3, 4, 5]
19. How can you use array iteration methods to transform an array of objects?
Answer: You can use map
to transform an array of objects by applying a function that changes the structure or properties of each object.
const users = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
];
const userNames = users.map(user => user.name);
console.log(userNames); // ['Alice', 'Bob']
20. Explain the lastIndexOf
method.
Answer: The lastIndexOf
method returns the last index at which a given element can be found in the array, or -1
if it is not present. It searches backwards from the end to the beginning of the array.