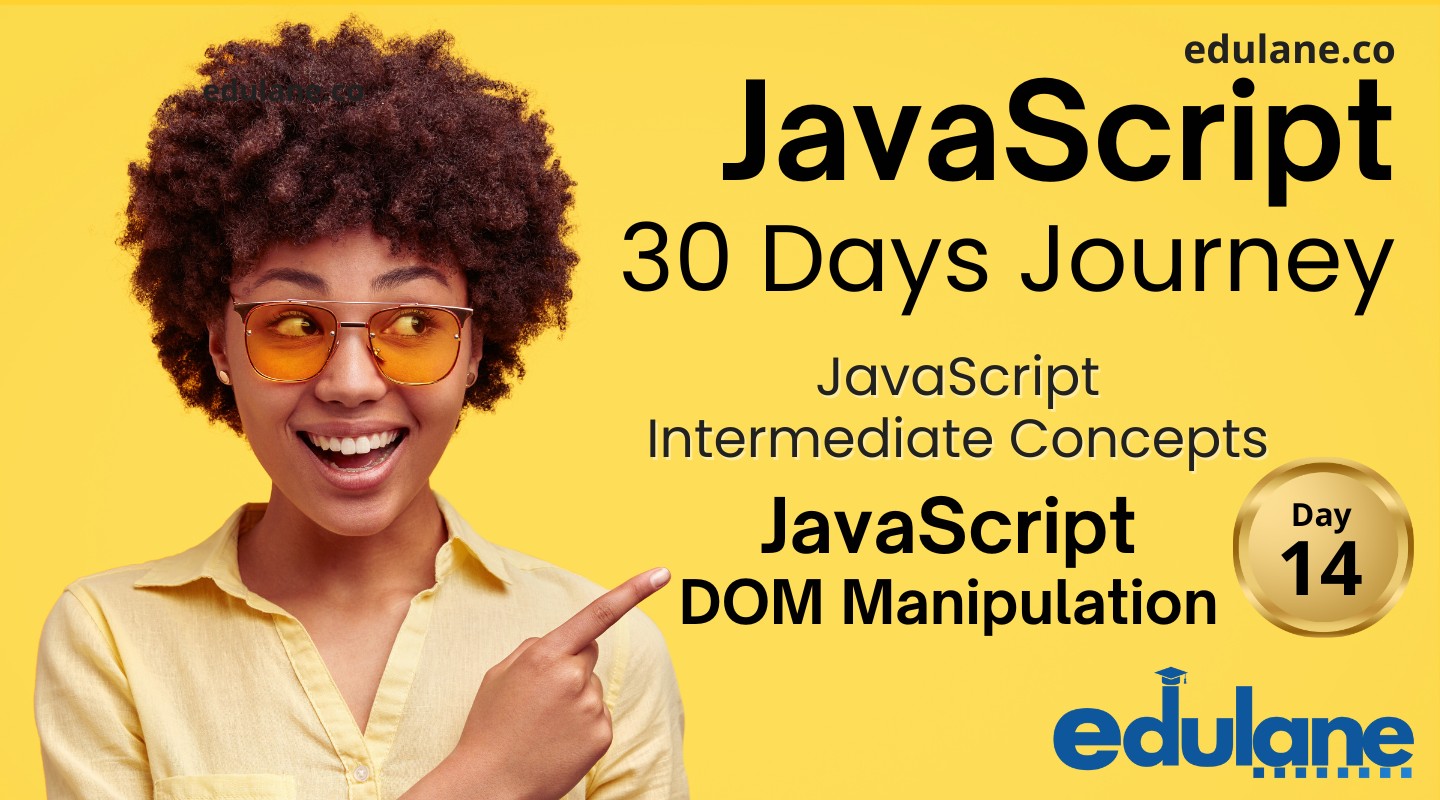
Detailed Guide on JavaScript DOM Manipulation
Table Of Contents
Section | Description |
1. Understanding the DOM | Introduction to the Document Object Model (DOM) and its importance in web development. |
2. Beginner Level: Selecting Elements | Basics of selecting elements using JavaScript. |
2.1 Example 1: Selecting Elements | Example demonstrating how to select elements by ID, class, and tag name. |
3. Intermediate Level: Modifying Elements | Techniques for modifying the content and style of elements. |
3.1 Example 2: Modifying Element Content and Style | Example showing how to change text content and apply styles to elements. |
4. Advanced Level: Adding and Removing Elements | Methods for creating and removing elements dynamically. |
4. Advanced Level: Adding and Removing Elements | Methods for creating and removing elements dynamically. |
4.1 Example 3: Creating and Removing Elements | Example illustrating how to add and remove elements from the DOM. |
5. Complex Level: Event Handling and Advanced DOM Manipulation | Advanced techniques for handling events and manipulating the DOM. |
5.1 Example 4: Event Listeners and Dynamic Content | Example showing how to use event listeners and dynamically update content. |
6. Best Practices for DOM Manipulation | Guidelines for efficient and maintainable DOM manipulation. |
7. Conclusion | Summary of the key points and final thoughts on DOM manipulation. |
1. Understanding the DOM
The DOM is a tree-like structure representing the content of a webpage. Each element on the page is a node in this tree, and JavaScript provides a variety of methods to interact with these nodes.
2. Beginner Level: Selecting Elements
The first step in DOM manipulation is selecting elements.
Example 1: Selecting Elements
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<h1 id="title">Hello, World!</h1>
<p class="description">This is a simple paragraph.</p>
<button onclick="changeTitle()">Change Title</button>
<script src="app.js"></script>
</body>
</html>
JavaScript (app.js):
// Selecting an element by ID
const title = document.getElementById('title');
// Selecting elements by class name
const description = document.querySelector('.description');
// Selecting elements by tag name
const paragraphs = document.getElementsByTagName('p');
Output:
When the webpage loads, it will display the h1
and p
elements. The JavaScript code selects these elements but doesn’t alter them yet.
Intermediate Level: Modifying Elements
Example 2: Modifying Element Content and Style
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<h1 id="title">Hello, World!</h1>
<p class="description">This is a simple paragraph.</p>
<button onclick="changeTitle()">Change Title</button>
<script src="app.js"></script>
</body>
</html>
JavaScript (app.js):
// Function to change the title's text and style
function changeTitle() {
const title = document.getElementById('title');
title.textContent = 'Hello, JavaScript!';
title.style.color = 'blue';
title.style.fontSize = '24px';
}
Output:
Clicking the button will change the h1
text to “Hello, JavaScript!” and modify its color and font size.
Advanced Level: Adding and Removing Elements
Example 3: Creating and Removing Elements
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<div id="container">
<h1 id="title">Hello, World!</h1>
<p class="description">This is a simple paragraph.</p>
</div>
<button onclick="addElement()">Add Element</button>
<button onclick="removeElement()">Remove Element</button>
<script src="app.js"></script>
</body>
</html>
JavaScript (app.js):
// Function to add a new paragraph
function addElement() {
const container = document.getElementById('container');
const newParagraph = document.createElement('p');
newParagraph.textContent = 'This is a new paragraph.';
container.appendChild(newParagraph);
}
// Function to remove the last paragraph
function removeElement() {
const container = document.getElementById('container');
const lastParagraph = container.querySelector('p:last-child');
if (lastParagraph) {
container.removeChild(lastParagraph);
}
}
Output:
Clicking “Add Element” adds a new paragraph to the div#container
. Clicking “Remove Element” removes the last paragraph.
Complex Level: Event Handling and Advanced DOM Manipulation
Example 4: Event Listeners and Dynamic Content
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<div id="container">
<h1 id="title">Hello, World!</h1>
<ul id="list"></ul>
<input type="text" id="itemInput" placeholder="Add item">
<button id="addItemBtn">Add Item</button>
</div>
<script src="app.js"></script>
</body>
</html>
JavaScript (app.js):
document.getElementById('addItemBtn').addEventListener('click', function() {
const itemInput = document.getElementById('itemInput');
const newItem = itemInput.value;
if (newItem.trim() !== '') {
const list = document.getElementById('list');
const listItem = document.createElement('li');
listItem.textContent = newItem;
list.appendChild(listItem);
itemInput.value = ''; // Clear the input
}
});
Output:
Entering text in the input field and clicking “Add Item” adds a new list item to the unordered list.
6. Best Practices for DOM Manipulation
1. Minimize Reflows and Repaints:
- Batch DOM read/write operations to minimize reflows and repaints for better performance.
// Bad practice
element.style.width = '100px';
element.style.height = '100px';
// Better practice
element.style.cssText = 'width: 100px; height: 100px;';
2. Use Event Delegation:
- Attach a single event listener to a parent element to handle events for multiple child elements.
document.getElementById('container').addEventListener('click', function(event) {
if (event.target.tagName === 'BUTTON') {
// Handle button click
}
});
3. Avoid Inline JavaScript:
- Separate JavaScript from HTML for maintainability.
<!-- Instead of this: -->
<button onclick="changeTitle()">Change Title</button>
<!-- Use this: -->
<button id="changeTitleBtn">Change Title</button>
<script>
document.getElementById('changeTitleBtn').addEventListener('click', changeTitle);
</script>
4. Cache DOM Selections:
- Store references to frequently accessed elements in variables.
const container = document.getElementById('container');
7. Conclusion
Understanding and manipulating the DOM is essential for creating interactive web applications. By mastering these concepts, you can create dynamic and responsive user interfaces. Remember to follow best practices for performance and maintainability.
Example 2: Accessing and Logging the Document Object
HTML:
<!DOCTYPE html>
<html>
<head>
<title>DOM Example</title>
</head>
<body>
<h1>Document Object</h1>
<script>
console.log(document.title); // Outputs: DOM Example
</script>
</body>
</html>
2. Accessing DOM Elements
2.1 Using getElementById
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<div id="myDiv">Hello World</div>
<script>
const element = document.getElementById('myDiv');
console.log(element); // Outputs: <div id="myDiv">Hello World</div>
</script>
</body>
</html>
Example 1: Changing Text Content
<!DOCTYPE html>
<html>
<head>
<title>getElementById Example</title>
</head>
<body>
<div id="greeting">Hello World</div>
<script>
const element = document.getElementById('greeting');
element.innerText = 'Hello, JavaScript!';
</script>
</body>
</html>
Example 2: Changing Style
<!DOCTYPE html>
<html>
<head>
<title>getElementById Example</title>
</head>
<body>
<div id="myDiv">Styled Text</div>
<script>
const element = document.getElementById('myDiv');
element.style.color = 'blue';
</script>
</body>
</html>
Example 3: Setting an Attribute
<!DOCTYPE html>
<html>
<head>
<title>getElementById Example</title>
</head>
<body>
<img id="myImage" src="oldImage.jpg" alt="Old Image">
<script>
const image = document.getElementById('myImage');
image.setAttribute('alt', 'Updated Image');
</script>
</body>
</html>
2.2 Using getElementsByClassName
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<div class="myClass">Hello</div>
<div class="myClass">World</div>
<script>
const elements = document.getElementsByClassName('myClass');
console.log(elements); // Outputs: HTMLCollection(2) [div.myClass, div.myClass]
</script>
</body>
</html>
Example 1: Changing Multiple Elements’ Text
<!DOCTYPE html>
<html>
<head>
<title>getElementsByClassName Example</title>
</head>
<body>
<div class="myClass">Hello</div>
<div class="myClass">World</div>
<script>
const elements = document.getElementsByClassName('myClass');
for (let i = 0; i < elements.length; i++) {
elements[i].innerText = 'Changed';
}
</script>
</body>
</html>
Example 2: Changing Multiple Elements’ Style
<!DOCTYPE html>
<html>
<head>
<title>getElementsByClassName Example</title>
</head>
<body>
<div class="myClass">Hello</div>
<div class="myClass">World</div>
<script>
const elements = document.getElementsByClassName('myClass');
for (let i = 0; i < elements.length; i++) {
elements[i].style.color = 'green';
}
</script>
</body>
</html>
Example 3: Changing Multiple Elements’ Attributes
<!DOCTYPE html>
<html>
<head>
<title>getElementsByClassName Example</title>
</head>
<body>
<img class="myImage" src="oldImage1.jpg" alt="Old Image 1">
<img class="myImage" src="oldImage2.jpg" alt="Old Image 2">
<script>
const images = document.getElementsByClassName('myImage');
for (let i = 0; i < images.length; i++) {
images[i].src = 'newImage.jpg';
}
</script>
</body>
</html>
2.3 Using querySelector
and querySelectorAll
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<div class="myClass">Hello</div>
<div class="myClass">World</div>
<script>
const element = document.querySelector('.myClass');
console.log(element); // Outputs: <div class="myClass">Hello</div>
const elements = document.querySelectorAll('.myClass');
console.log(elements); // Outputs: NodeList(2) [div.myClass, div.myClass]
</script>
</body>
</html>
Example 1: Changing the First Element’s Text
<!DOCTYPE html>
<html>
<head>
<title>querySelector Example</title>
</head>
<body>
<div class="myClass">Hello</div>
<div class="myClass">World</div>
<script>
const element = document.querySelector('.myClass');
element.innerText = 'First Element';
</script>
</body>
</html>
Example 2: Changing All Elements’ Text
<!DOCTYPE html>
<html>
<head>
<title>querySelectorAll Example</title>
</head>
<body>
<div class="myClass">Hello</div>
<div class="myClass">World</div>
<script>
const elements = document.querySelectorAll('.myClass');
elements.forEach(element => {
element.innerText = 'Changed';
});
</script>
</body>
</html>
Example 3: Changing Multiple Elements’ Style
<!DOCTYPE html>
<html>
<head>
<title>querySelectorAll Example</title>
</head>
<body>
<div class="myClass">Hello</div>
<div class="myClass">World</div>
<script>
const elements = document.querySelectorAll('.myClass');
elements.forEach(element => {
element.style.color = 'purple';
});
</script>
</body>
</html>
3. Modifying DOM Elements
3.1 Changing Inner Content
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<div id="myDiv">Hello World</div>
<button onclick="changeContent()">Change Content</button>
<script>
function changeContent() {
const element = document.getElementById('myDiv');
element.innerHTML = 'Content Changed!';
}
</script>
</body>
</html>
Example 1: Using innerHTML
<!DOCTYPE html>
<html>
<head>
<title>innerHTML Example</title>
</head>
<body>
<div id="content">Original Content</div>
<button onclick="changeContent()">Change Content</button>
<script>
function changeContent() {
document.getElementById('content').innerHTML = '<p>New Content</p>';
}
</script>
</body>
</html>
Example 2: Using innerText
<!DOCTYPE html>
<html>
<head>
<title>innerText Example</title>
</head>
<body>
<div id="content">Original Content</div>
<button onclick="changeContent()">Change Content</button>
<script>
function changeContent() {
document.getElementById('content').innerText = 'New Text Content';
}
</script>
</body>
</html>
Example 3: Using textContent
<!DOCTYPE html>
<html>
<head>
<title>textContent Example</title>
</head>
<body>
<div id="content">Original Content</div>
<button onclick="changeContent()">Change Content</button>
<script>
function changeContent() {
document.getElementById('content').textContent = 'New Text Content';
}
</script>
</body>
</html>
3.2 Changing Attributes
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<img id="myImage" src="oldImage.jpg" alt="Old Image">
<button onclick="changeImage()">Change Image</button>
<script>
function changeImage() {
const image = document.getElementById('myImage');
image.src = 'newImage.jpg';
image.alt = 'New Image';
}
</script>
</body>
</html>
Example 1: Setting an Attribute
<!DOCTYPE html>
<html>
<head>
<title>setAttribute Example</title>
</head>
<body>
<img id="myImage" src="oldImage.jpg" alt="Old Image">
<button onclick="changeImage()">Change Image</button>
<script>
function changeImage() {
const image = document.getElementById('myImage');
image.setAttribute('src', 'newImage.jpg');
}
</script>
</body>
</html>
Example 2: Getting an Attribute
<!DOCTYPE html>
<html>
<head>
<title>getAttribute Example</title>
</head>
<body>
<img id="myImage" src="image.jpg" alt="An Image">
<button onclick="logAlt()">Log Alt</button>
<script>
function logAlt() {
const image = document.getElementById('myImage');
console.log(image.getAttribute('alt')); // Outputs: An Image
}
</script>
</body>
</html>
Example 3: Removing an Attribute
<!DOCTYPE html>
<html>
<head>
<title>removeAttribute Example</title>
</head>
<body>
<img id="myImage" src="image.jpg" alt="An Image">
<button onclick="removeAlt()">Remove Alt</button>
<script>
function removeAlt() {
const image = document.getElementById('myImage');
image.removeAttribute('alt');
}
</script>
</body>
</html>
3.3 Changing Styles
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<div id="myDiv">Styled Text</div>
<button onclick="changeStyle()">Change Style</button>
<script>
function changeStyle() {
const element = document.getElementById('myDiv');
element.style.color = 'red';
element.style.fontSize = '20px';
}
</script>
</body>
</html>
Example 1: Setting a Single Style Property
<!DOCTYPE html>
<html>
<head>
<title>Style Example</title>
</head>
<body>
<div id="styledDiv">This is a div</div>
<button onclick="changeStyle()">Change Style</button>
<script>
function changeStyle() {
const element = document.getElementById('styledDiv');
element.style.backgroundColor = 'lightblue';
}
</script>
</body>
</html>
Example 2: Setting Multiple Style Properties
<!DOCTYPE html>
<html>
<head>
<title>Style Example</title>
</head>
<body>
<div id="styledDiv">This is a div</div>
<button onclick="changeStyle()">Change Style</button>
<script>
function changeStyle() {
const element = document.getElementById('styledDiv');
element.style.color = 'white';
element.style.backgroundColor = 'black';
element.style.padding = '10px';
}
</script>
</body>
</html>
Example 3: Toggling a Style Class
<!DOCTYPE html>
<html>
<head>
<title>Class Example</title>
<style>
.highlight {
background-color: yellow;
font-weight: bold;
}
</style>
</head>
<body>
<div id="myDiv">Toggle Class Example</div>
<button onclick="toggleClass()">Toggle Class</button>
<script>
function toggleClass() {
const element = document.getElementById('myDiv');
element.classList.toggle('highlight');
}
</script>
</body>
</html>
4. Creating and Removing Elements
4.1 Creating Elements
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<div id="parentDiv"></div>
<button onclick="addElement()">Add Element</button>
<script>
function addElement() {
const parent = document.getElementById('parentDiv');
const newElement = document.createElement('p');
newElement.innerHTML = 'This is a new paragraph';
parent.appendChild(newElement);
}
</script>
</body>
</html>
Example 1: Adding a New Paragraph
<!DOCTYPE html>
<html>
<head>
<title>Create Element Example</title>
</head>
<body>
<div id="parentDiv"></div>
<button onclick="addParagraph()">Add Paragraph</button>
<script>
function addParagraph() {
const parent = document.getElementById('parentDiv');
const newParagraph = document.createElement('p');
newParagraph.innerHTML = 'This is a new paragraph';
parent.appendChild(newParagraph);
}
</script>
</body>
</html>
Example 2: Adding Multiple Elements
<!DOCTYPE html>
<html>
<head>
<title>Create Element Example</title>
</head>
<body>
<div id="parentDiv"></div>
<button onclick="addElements()">Add Elements</button>
<script>
function addElements() {
const parent = document.getElementById('parentDiv');
for (let i = 1; i <= 3; i++) {
const newElement = document.createElement('p');
newElement.innerHTML = `Paragraph ${i}`;
parent.appendChild(newElement);
}
}
</script>
</body>
</html>
Example 3: Adding an Image
<!DOCTYPE html>
<html>
<head>
<title>Create Element Example</title>
</head>
<body>
<div id="parentDiv"></div>
<button onclick="addImage()">Add Image</button>
<script>
function addImage() {
const parent = document.getElementById('parentDiv');
const newImage = document.createElement('img');
newImage.src = 'image.jpg';
newImage.alt = 'New Image';
parent.appendChild(newImage);
}
</script>
</body>
</html>
4.2 Removing Elements
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<div id="parentDiv">
<p id="myParagraph">This is a paragraph</p>
</div>
<button onclick="removeElement()">Remove Element</button>
<script>
function removeElement() {
const element = document.getElementById('myParagraph');
element.remove();
}
</script>
</body>
</html>
Example 1: Removing a Specific Element
<!DOCTYPE html>
<html>
<head>
<title>Remove Element Example</title>
</head>
<body>
<div id="parentDiv">
<p id="myParagraph">This is a paragraph</p>
</div>
<button onclick="removeElement()">Remove Element</button>
<script>
function removeElement() {
const element = document.getElementById('myParagraph');
element.remove();
}
</script>
</body>
</html>
Example 2: Removing All Child Elements
<!DOCTYPE html>
<html>
<head>
<title>Remove Element Example</title>
</head>
<body>
<div id="parentDiv">
<p>This is a paragraph</p>
<p>This is another paragraph</p>
</div>
<button onclick="removeAllElements()">Remove All Elements</button>
<script>
function removeAllElements() {
const parent = document.getElementById('parentDiv');
while (parent.firstChild) {
parent.removeChild(parent.firstChild);
}
}
</script>
</body>
</html>
Example 3: Removing an Element on Click
<!DOCTYPE html>
<html>
<head>
<title>Remove Element Example</title>
</head>
<body>
<div id="parentDiv">
<p onclick="this.remove()">Click to remove this paragraph</p>
<p onclick="this.remove()">Click to remove this paragraph</p>
</div>
</body>
</html>
5. Event Handling
5.1 Adding Event Listeners
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
const button = document.getElementById('myButton');
button.addEventListener('click', function() {
alert('Button was clicked!');
});
</script>
</body>
</html>
Example 1: Click Event
<!DOCTYPE html>
<html>
<head>
<title>Event Listener Example</title>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
const button = document.getElementById('myButton');
button.addEventListener('click', function() {
alert('Button was clicked!');
});
</script>
</body>
</html>
Example 2: Mouseover Event
<!DOCTYPE html>
<html>
<head>
<title>Event Listener Example</title>
</head>
<body>
<div id="myDiv">Hover over me</div>
<script>
const div = document.getElementById('myDiv');
div.addEventListener('mouseover', function() {
div.style.color = 'blue';
});
</script>
</body>
</html>
Example 3: Keydown Event
<!DOCTYPE html>
<html>
<head>
<title>Event Listener Example</title>
</head>
<body>
<input type="text" id="myInput">
<script>
const input = document.getElementById('myInput');
input.addEventListener('keydown', function(event) {
console.log(`Key pressed: ${event.key}`);
});
</script>
</body>
</html>
5.2 Removing Event Listeners
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<body>
<button id="myButton">Click Me</button>
<button onclick="removeEvent()">Remove Event Listener</button>
<script>
const button = document.getElementById('myButton');
function showAlert() {
alert('Button was clicked!');
}
button.addEventListener('click', showAlert);
function removeEvent() {
button.removeEventListener('click', showAlert);
}
</script>
</body>
</html>
Example 1: Removing a Click Event Listener
<!DOCTYPE html>
<html>
<head>
<title>Remove Event Listener Example</title>
</head>
<body>
<button id="myButton">Click Me</button>
<button onclick="removeEvent()">Remove Event Listener</button>
<script>
const button = document.getElementById('myButton');
function showAlert() {
alert('Button was clicked!');
}
button.addEventListener('click', showAlert);
function removeEvent() {
button.removeEventListener('click', showAlert);
}
</script>
</body>
</html>
Example 2: Removing a Mouseover Event Listener
<!DOCTYPE html>
<html>
<head>
<title>Remove Event Listener Example</title>
</head>
<body>
<div id="myDiv">Hover over me</div>
<button onclick="removeEvent()">Remove Mouseover Event</button>
<script>
const div = document.getElementById('myDiv');
function changeColor() {
div.style.color = 'blue';
}
div.addEventListener('mouseover', changeColor);
function removeEvent() {
div.removeEventListener('mouseover', changeColor);
}
</script>
</body>
</html>
Example 3: Removing a Keydown Event Listener
<!DOCTYPE html>
<html>
<head>
<title>Remove Event Listener Example</title>
</head>
<body>
<input type="text" id="myInput">
<button onclick="removeEvent()">Remove Keydown Event</button>
<script>
const input = document.getElementById('myInput');
function logKey(event) {
console.log(`Key pressed: ${event.key}`);
}
input.addEventListener('keydown', logKey);
function removeEvent() {
input.removeEventListener('keydown', logKey);
}
</script>
</body>
</html>
6. Best Practices
- Separation of Concerns: Keep your HTML, CSS, and JavaScript separate. Use external files for better maintainability.
- Avoid Inline Styles and Event Handlers: Use
addEventListener
and style classes instead. - Cache DOM References: Store references to DOM elements in variables to avoid repeated querying.
- Use Delegation for Dynamic Content: Use event delegation to handle events on dynamically added elements efficiently.
- Clean Up: Remove event listeners and DOM elements when they are no longer needed to prevent memory leaks.
7. Example: Dynamic List
HTML
<!DOCTYPE html>
<html>
<head>
<title>Dynamic List</title>
<style>
.list-item {
margin: 5px 0;
}
</style>
</head>
<body>
<input type="text" id="itemInput" placeholder="Add an item">
<button onclick="addItem()">Add Item</button>
<ul id="itemList"></ul>
<script src="script.js"></script>
</body>
</html>
JavaScript (script.js
)
document.addEventListener('DOMContentLoaded', function() {
const input = document.getElementById('itemInput');
const list = document.getElementById('itemList');
document.querySelector('button').addEventListener('click', addItem);
function addItem() {
const value = input.value.trim();
if (value !== '') {
const li = document.createElement('li');
li.className = 'list-item';
li.textContent = value;
list.appendChild(li);
input.value = '';
}
}
});
Example 1: Simple Todo List
<!DOCTYPE html>
<html>
<head>
<title>Todo List Example</title>
</head>
<body>
<h1>Todo List</h1>
<input type="text" id="newTodo" placeholder="Add a new todo">
<button onclick="addTodo()">Add</button>
<ul id="todoList"></ul>
<script>
const todoList = document.getElementById('todoList');
function addTodo() {
const newTodoText = document.getElementById('newTodo').value;
if (newTodoText) {
const newTodoItem = document.createElement('li');
newTodoItem.innerText = newTodoText;
newTodoItem.addEventListener('click', function() {
newTodoItem.remove();
});
todoList.appendChild(newTodoItem);
document.getElementById('newTodo').value = '';
}
}
</script>
</body>
</html>
Example 2: Real-time Character Count
<!DOCTYPE html>
<html>
<head>
<title>Character Count Example</title>
</head>
<body>
<h1>Character Count</h1>
<textarea id="textInput" rows="4" cols="50"></textarea>
<p>Characters: <span id="charCount">0</span></p>
<script>
const textInput = document.getElementById('textInput');
const charCount = document.getElementById('charCount');
textInput.addEventListener('input', function() {
charCount.innerText = textInput.value.length;
});
</script>
</body>
</html>
Explanation
- HTML: We have an input field, a button, and an unordered list.
- JavaScript: We wait for the DOM content to load before adding event listeners. When the button is clicked, the
addItem
function is called. addItem
Function: It takes the value from the input, trims any whitespace, and if it’s not empty, creates a new list item, sets its text content, and appends it to the list. Finally, it clears the input field.
Output
When you type something into the input field and click the “Add Item” button, a new list item appears in the unordered list with the text you entered.
By following these steps and best practices, you can effectively manipulate the DOM using JavaScript, creating dynamic and interactive web pages.
Interview Questions
Here are 20 interview questions and answers focused on JavaScript DOM manipulation:
Basic Questions
1.What is the DOM?
Answer:
The DOM (Document Object Model) is a programming interface for HTML and XML documents. It represents the page structure as a tree of objects so that programs can dynamically interact with and modify the content, structure, and style of the document.
2. How do you select an element by its ID in JavaScript?
Answer:
You can select an element by its ID using the document.getElementById()
method.
const element = document.getElementById('elementId');
3. How do you select elements by their class name?
Answer:
You can select elements by their class name using the document.getElementsByClassName()
method.
const elements = document.getElementsByClassName('className');
4. How do you select elements by their tag name?
Answer:
You can select elements by their tag name using the document.getElementsByTagName()
method.
const elements = document.getElementsByTagName('tagName');
5. How can you select elements using a CSS selector?
Answer:
You can select elements using a CSS selector with document.querySelector()
or document.querySelectorAll()
.
const element = document.querySelector('.className'); // Single element
const elements = document.querySelectorAll('.className'); // NodeList of elements
Intermediate Questions
6. What is the difference between innerHTML
, innerText
, and textContent
?
Answer:
innerHTML
: Gets or sets the HTML content of an element.innerText
: Gets or sets the text content of an element, taking into account CSS styling (e.g.,display: none
).textContent
: Gets or sets the text content of an element, ignoring CSS styling.
7. How do you add a new element to the DOM?
Answer:
You create a new element using document.createElement()
and append it using appendChild()
.
const newElement = document.createElement('div');
const parentElement = document.getElementById('parent');
parentElement.appendChild(newElement);
8. How can you remove an element from the DOM?
Answer:
You can remove an element using the remove()
method or removeChild()
method.
const element = document.getElementById('elementId');
element.remove();
// or
const parentElement = document.getElementById('parent');
const childElement = document.getElementById('child');
parentElement.removeChild(childElement);
9. How do you add an event listener to an element?
Answer:
You can add an event listener using the addEventListener()
method.
const button = document.getElementById('buttonId');
button.addEventListener('click', function() {
alert('Button was clicked!');
});
10. What is event delegation and why is it useful?
Answer:
Event delegation is a technique where a single event listener is added to a parent element to manage events for its child elements. It’s useful for handling events on dynamically added elements and for improving performance by reducing the number of event listeners.
Advanced Questions
11. How do you clone an element in the DOM?
Answer:
You can clone an element using the cloneNode()
method.
const original = document.getElementById('original');
const clone = original.cloneNode(true); // true for deep clone
document.body.appendChild(clone);
12. How do you get and set the value of an input field?
Answer:
You can get and set the value of an input field using the value
property.
const input = document.getElementById('inputId');
const value = input.value; // Get value
input.value = 'newValue'; // Set value
13. How do you modify the CSS styles of an element?
Answer:
You can modify the CSS styles using the style
property
const element = document.getElementById('elementId');
element.style.color = 'red';
element.style.backgroundColor = 'blue';
14. How do you get and set attributes of an element?
Answer:
You can get and set attributes using getAttribute()
and setAttribute()
.
const element = document.getElementById('elementId');
const value = element.getAttribute('data-value'); // Get attribute
element.setAttribute('data-value', 'newValue'); // Set attribute
15. How do you manipulate classes of an element?
Answer: You can manipulate classes using the classList
property.
const element = document.getElementById('elementId');
element.classList.add('newClass');
element.classList.remove('oldClass');
element.classList.toggle('toggleClass');
Expert Questions
16. What is the DOMContentLoaded
event and how is it different from the load
event?
Answer:
The DOMContentLoaded
event fires when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading. The load
event fires when the entire page, including all dependent resources, has finished loading.
17. How can you prevent the default action of an event?
Answer:
You can prevent the default action using the preventDefault()
method.
const link = document.getElementById('linkId');
link.addEventListener('click', function(event) {
event.preventDefault();
alert('Default action prevented!');
});
18. How do you stop event propagation?
Answer:
You can stop event propagation using the stopPropagation()
method.
const button = document.getElementById('buttonId');
button.addEventListener('click', function(event) {
event.stopPropagation();
alert('Event propagation stopped!');
});
19. How do you handle events for dynamically added elements?
Answer:
You can handle events for dynamically added elements using event delegation. Attach the event listener to a common ancestor and check the event target.
const parent = document.getElementById('parentId');
parent.addEventListener('click', function(event) {
if (event.target && event.target.matches('button.dynamic')) {
alert('Dynamically added button clicked!');
}
});
20. What are the nextSibling
and previousSibling
properties?
Answer:
The nextSibling
property returns the next sibling node of the specified node, and the previousSibling
property returns the previous sibling node. These properties include text nodes (whitespace)
const element = document.getElementById('elementId');
const nextSibling = element.nextSibling;
const previousSibling = element.previousSibling;
These questions cover a range of topics from basic to advanced DOM manipulation, providing a comprehensive overview of essential concepts and techniques.