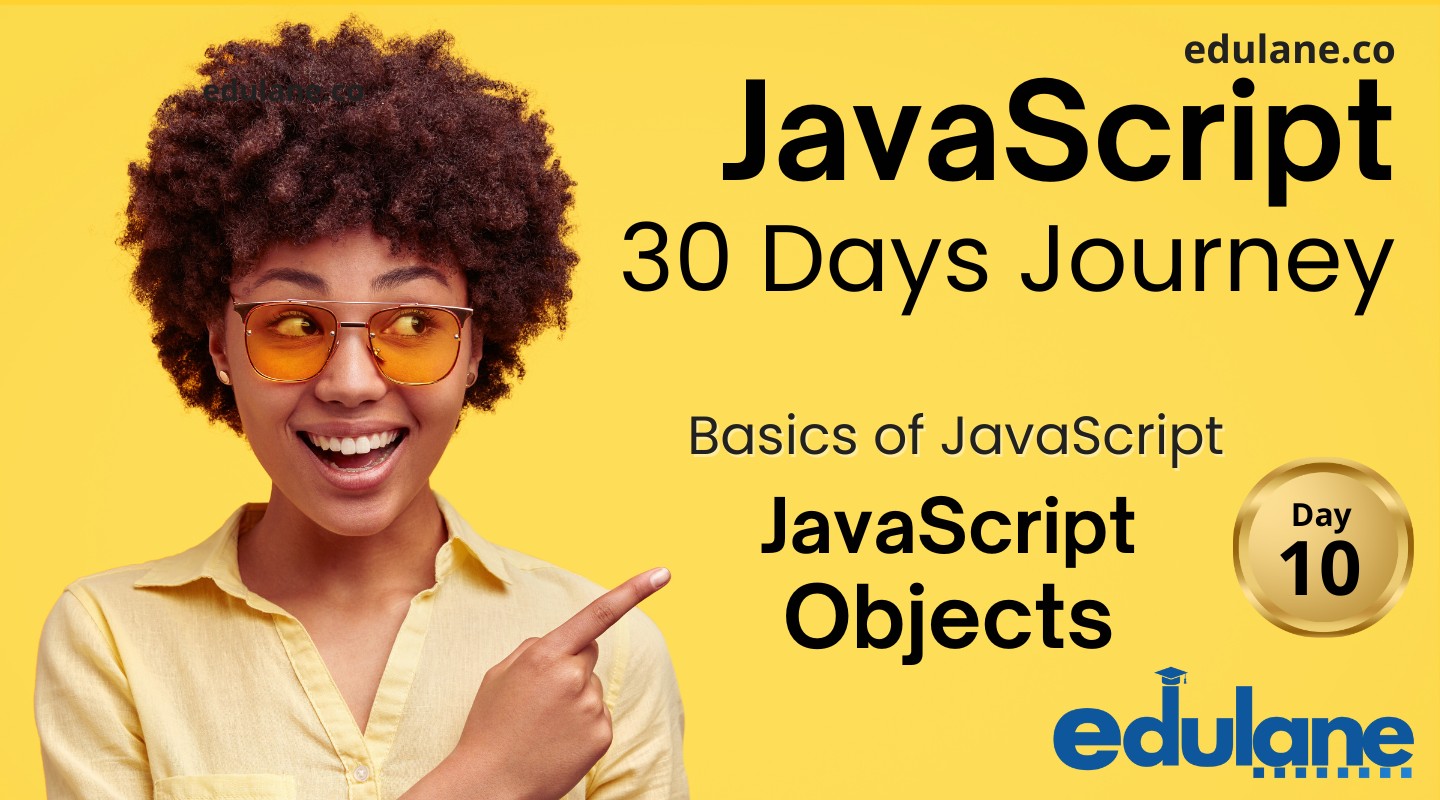
Understand JavaScript objects with Edulane. This detailed guide takes you through the basics of object creation, properties, and methods, progressing to advanced concepts and practical applications, enhancing your web development expertise.
Introduction to JavaScript Objects
JavaScript objects are a fundamental data structure used to store collections of data and more complex entities. Objects consist of key-value pairs, where the keys are strings (or symbols) and the values can be any data type, including other objects.
Basic JavaScript Objects
In JavaScript, an object is a collection of properties, where each property is a key-value pair. The key is a string, and the value can be any data type, including numbers, strings, arrays, or even other objects.
// Using camelCase for property names
let person = {
firstName: "John",
age: 30,
isEmployed: true
};
console.log(person.firstName); // Output: John
console.log(person.age); // Output: 30
console.log(person.isEmployed); // Output: true
Explanation:
- Key-Value Pairs: The object
person
has three properties:firstName
,age
, andisEmployed
. - Accessing Properties: Properties are accessed using dot notation (e.g.,
person.firstName
).
Nested Objects
Objects can contain other objects as values. This allows for creating complex data structures.
let employee = {
name: "Jane",
age: 28,
position: {
title: "Software Engineer",
department: "IT"
}
};
console.log(employee.position.title); // Output: Software Engineer
console.log(employee.position.department); // Output: IT
Explanation:
- Nested Object: The
position
property itself is an object withtitle
anddepartment
. - Accessing Nested Properties: Use dot notation to access properties of nested objects (e.g.,
employee.position.title
).
Methods in Objects
Objects can also contain methods, which are functions defined as properties of the object.
let calculator = {
add(a, b) {
return a + b;
},
subtract(a, b) {
return a - b;
}
};
console.log(calculator.add(5, 3)); // Output: 8
console.log(calculator.subtract(5, 3)); // Output: 2
Explanation:
- Method Definition: Methods are defined using the shorthand syntax (e.g.,
add(a, b)
). - Calling Methods: Methods are called like regular properties (e.g.,
calculator.add(5, 3)
).
The this Keyword
The this
keyword refers to the object that is currently executing the code. It allows methods to access other properties of the same object.
let car = {
brand: "Toyota",
model: "Camry",
fullName() {
return `${this.brand} ${this.model}`;
}
};
console.log(car.fullName()); // Output: Toyota Camry
Explanation:
this
Context: Inside thefullName
method,this
refers to thecar
object.- Using
this
:this.brand
andthis.model
access properties of thecar
object.
ES6 Classes
ES6 introduced a new syntax for creating classes and handling inheritance, providing a more structured and readable approach compared to traditional constructor functions and prototypes. Below is a detailed explanation of ES6 classes, including their syntax, features, and best practices.
1. Class Definition
ES6 classes use a simpler and more intuitive syntax for defining and working with objects compared to traditional function-based prototypes. Classes encapsulate data and methods in a clear, structured manner.
// Syntax
class ClassName {
constructor(parameters) {
// Initialization code
}
methodName() {
// Method code
}
}
// Example: Basic Class
// Define a class named Person
class Person {
constructor(name, age) {
this.name = name; // Instance property
this.age = age; // Instance property
}
// Instance method
greet() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
// Create an instance of Person
const person1 = new Person("Alice", 30);
// Call the greet method
person1.greet(); // Output: Hello, my name is Alice and I am 30 years old.
Explanation:
- Class Declaration:
class Person
declares a new class namedPerson
. - Constructor: The
constructor
method initializes properties (name
andage
). - Method:
greet
is an instance method that prints a message using the instance properties.
2. Inheritance with ES6 Classes
ES6 classes support inheritance, allowing you to create a new class that extends an existing class. This is done using the extends
keyword, and the super
keyword is used to call methods from the parent class.
// Syntax
class ParentClass {
constructor(parameters) {
// Initialization code
}
parentMethod() {
// Method code
}
}
class ChildClass extends ParentClass {
constructor(parameters) {
super(parameters); // Call parent class constructor
// Additional initialization code
}
childMethod() {
// Method code
}
}
// Example: Class Inheritance
// Parent class
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
// Child class
class Dog extends Animal {
constructor(name) {
super(name); // Call parent class constructor
}
speak() {
console.log(`${this.name} barks.`);
}
}
// Create instances
const genericAnimal = new Animal("Generic Animal");
const dog = new Dog("Rex");
// Call methods
genericAnimal.speak(); // Output: Generic Animal makes a noise.
dog.speak(); // Output: Rex barks.
/*
Explanation
1. Inheritance: Dog extends Animal, meaning Dog inherits properties and methods from Animal.
super Keyword: super(name) calls the Animal constructor to initialize the name property in the Dog class.
2. Method Overriding: The speak method in Dog overrides the speak method in Animal.
*/
3. Getters and Setters
Getters and setters allow you to define how properties are accessed and modified. They provide a way to encapsulate and validate property access and assignment.
// Syntax
class MyClass {
constructor(value) {
this._value = value;
}
// Getter
get value() {
return this._value;
}
// Setter
set value(newValue) {
if (newValue >= 0) {
this._value = newValue;
} else {
console.log("Value must be non-negative.");
}
}
}
// Example: Getters and Setters
class Rectangle {
constructor(width, height) {
this._width = width;
this._height = height;
}
// Getter for area
get area() {
return this._width * this._height;
}
// Setter for width
set width(newWidth) {
if (newWidth > 0) {
this._width = newWidth;
}
}
}
// Create an instance
const rect = new Rectangle(10, 5);
// Use getter
console.log(rect.area); // Output: 50
// Use setter
rect.width = 15;
console.log(rect.area); // Output: 75
/*
Explanation
1. Getter: get area() returns the computed area of the rectangle.
2. Setter: set width(newWidth) validates and updates the width of the rectangle.
*/
4. Static Methods and Properties
Static methods and properties belong to the class itself rather than instances of the class. They are used for utility functions or constants that are related to the class but do not operate on instance data.
// Syntax
class MyClass {
static staticMethod() {
// Static method code
}
static staticProperty = 'staticValue';
}
// Example: Static Methods and Properties
class MathUtils {
static add(a, b) {
return a + b;
}
static PI = 3.14159;
}
// Use static method
console.log(MathUtils.add(5, 3)); // Output: 8
// Use static property
console.log(MathUtils.PI); // Output: 3.14159
/*
Explanation:
1. Static Method: static add(a, b) is a static method that can be called directly on the class (MathUtils.add).
2. Static Property: static PI is a static property accessible on the class itself (MathUtils.PI).
*/
5. Private Fields and Methods
ES2022 introduced private fields and methods to classes. These are denoted by a #
prefix and are accessible only within the class that defines them.
// Syntax
class MyClass {
#privateField;
constructor(value) {
this.#privateField = value;
}
#privateMethod() {
console.log('This is a private method');
}
publicMethod() {
this.#privateMethod();
}
}
// Example: Private Fields and Methods
class Counter {
#count = 0; // Private field
increment() {
this.#count++;
}
getCount() {
return this.#count;
}
}
// Create an instance
const counter = new Counter();
counter.increment();
console.log(counter.getCount()); // Output: 1
// The following will result in an error
// console.log(counter.#count); // SyntaxError: Private field '#count' must be declared in an enclosing class
/*
Explanation:
1. Private Field: #count is a private field that is not accessible outside the class.
2. Private Method: #privateMethod() is a private method that can only be called within the class.
*/
Best Practices
Following best practices helps in writing maintainable and efficient code when using ES6 classes.
- Use Classes for Clear Structure: Use ES6 classes to create a clear and structured approach to object-oriented design.
- Encapsulation: Use private fields and methods to encapsulate and protect internal data.
- Static Methods: Use static methods for utility functions that do not depend on instance data.
- Consistent Naming: Use PascalCase for class names and camelCase for method and property names.
- Inheritance: Use inheritance to build upon existing functionality while keeping your code DRY (Don’t Repeat Yourself).
- Avoid Complex Inheritance Chains: Prefer composition over inheritance when dealing with complex relationships to avoid deeply nested and hard-to-maintain code.
Prototypes and Inheritance
JavaScript uses prototypes to implement inheritance. Understanding prototypes is crucial for mastering object-oriented programming in JavaScript. Below is a detailed explanation of prototypes and inheritance in JavaScript with clear examples and explanations.
1. Understanding Prototypes
Every JavaScript object has a prototype, which is another object from which it inherits properties and methods. When you try to access a property or method on an object, JavaScript first looks for it on the object itself. If it doesn’t find it, JavaScript looks up the prototype chain.
Key Concepts:
- Prototype: An object that provides properties and methods to another object.
- Prototype Chain: The chain of objects that JavaScript traverses when accessing properties and methods. It starts from the object itself and goes up through its prototype chain.
// Basic Prototype Usage
// Constructor function
function Animal(name) {
this.name = name;
}
// Prototype method
Animal.prototype.speak = function() {
console.log(`${this.name} makes a noise.`);
};
// Creating an instance
let dog = new Animal("Dog");
dog.speak(); // Output: Dog makes a noise.
Explanation:
- Constructor Function:
Animal
is a constructor function used to create instances ofAnimal
. - Prototype Method: The
speak
method is added toAnimal.prototype
, so all instances ofAnimal
inherit this method. - Instance: When
dog
is created, it inherits thespeak
method fromAnimal.prototype
.
Prototypal Inheritance
Prototypal inheritance allows one object to inherit properties and methods from another object. This is done by setting the prototype of one object to another object.
// Example: Prototypal Inheritance
// Constructor function
function Animal(name) {
this.name = name;
}
// Prototype method
Animal.prototype.speak = function() {
console.log(`${this.name} makes a noise.`);
};
// Constructor function for a specific type of animal
function Dog(name) {
// Call the parent constructor
Animal.call(this, name);
}
// Inherit from Animal
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
// Override the speak method
Dog.prototype.speak = function() {
console.log(`${this.name} barks.`);
};
// Creating an instance
let myDog = new Dog("Rex");
myDog.speak(); // Output: Rex barks.
Explanation:
- Constructor Function:
Animal
is the parent constructor function. - Child Constructor Function:
Dog
is a child constructor function that inherits fromAnimal
. - Inheritance Setup:
Dog.prototype
is set to an object created fromAnimal.prototype
, establishing inheritance. - Override Method: The
speak
method inDog.prototype
overrides the one inherited fromAnimal.prototype
.
ES6 Classes and Inheritance
ES6 introduces a class syntax that simplifies the creation of objects and inheritance. Classes provide a more intuitive and readable way to work with prototypes and inheritance.
// Example: ES6 Classes
// Parent class
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
// Child class
class Dog extends Animal {
constructor(name) {
super(name); // Call the parent constructor
}
speak() {
console.log(`${this.name} barks.`);
}
}
// Creating an instance
let myDog = new Dog("Rex");
myDog.speak(); // Output: Rex barks.
Explanation:
- Class Declaration:
Animal
andDog
are defined using theclass
keyword. - Inheritance:
Dog
inherits fromAnimal
using theextends
keyword. - Constructor:
super(name)
calls the parent class’s constructor. - Method Overriding: The
speak
method inDog
overrides the one inAnimal
.
Key Points to Remember
Prototype Chain
- Object Lookup: When a property or method is accessed, JavaScript looks up the prototype chain starting from the object itself.
Object.prototype
: At the end of the prototype chain isObject.prototype
, which is the root of all objects.
Creating Inheritance
- Constructor Functions: Use
Object.create
to set up inheritance. - ES6 Classes: Use
extends
andsuper
for a cleaner, more readable syntax.
Best Practices
- Avoid Modifying Built-in Prototypes: Modifying prototypes of built-in objects (like
Array.prototype
) can lead to unexpected behavior and conflicts. - Use Classes for Clarity: Use ES6 classes for a more intuitive and clear approach to inheritance.
Full Example: Combining Concepts
Here’s a comprehensive example that demonstrates prototypes and inheritance in both traditional and ES6 class syntax:
// Traditional Prototype-Based Inheritance
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(`${this.name} makes a noise.`);
};
function Dog(name) {
Animal.call(this, name);
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.speak = function() {
console.log(`${this.name} barks.`);
};
// Using ES6 Classes
class AnimalClass {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class DogClass extends AnimalClass {
constructor(name) {
super(name);
}
speak() {
console.log(`${this.name} barks.`);
}
}
// Create instances
let traditionalDog = new Dog("Rex");
let es6Dog = new DogClass("Max");
traditionalDog.speak(); // Output: Rex barks.
es6Dog.speak(); // Output: Max barks.
Explanation:
- Traditional Inheritance: Uses constructor functions and prototype chain setup.
- ES6 Classes: Provides a more modern and readable syntax for the same inheritance model.
- Instance Creation: Both traditional and ES6 class-based instances demonstrate how inheritance works.
Getters and Setters
Getters and setters are special methods that allow you to define how properties are accessed and modified.
class Person {
constructor(name, age) {
this._name = name;
this._age = age;
}
get name() {
return this._name;
}
set name(newName) {
this._name = newName;
}
get age() {
return this._age;
}
set age(newAge) {
if (newAge > 0) {
this._age = newAge;
}
}
}
let person = new Person("Alice", 25);
console.log(person.name); // Output: Alice
person.name = "Bob";
console.log(person.name); // Output: Bob
person.age = 30;
console.log(person.age); // Output: 30
Explanation:
- Getters:
name
andage
methods are defined as getters. - Setters:
name
andage
methods are defined as setters, allowing validation or transformation of values.
Best Practices
Best practices help in writing clean, maintainable, and efficient JavaScript code. Here are some recommended practices for working with objects:
Best Practices
- Use
const
for Object Declarations: Useconst
to declare objects when their reference does not need to change.
const person = { name: "Alice", age: 25 };
- Use Descriptive Key Names: Key names should be clear and descriptive to improve code readability.
let userProfile = {
firstName: "John",
lastName: "Doe",
isActive: true
};
- Avoid Deeply Nested Structures: Avoid creating deeply nested objects to reduce complexity and improve maintainability.
let userProfile = {
personalInfo: {
name: "Jane",
address: {
street: "123 Main St",
city: "Springfield"
}
},
preferences: {
theme: "dark"
}
};
- Use ES6 Classes for Complex Structures: Use ES6 classes for object creation and inheritance to leverage modern JavaScript features.
- Use Getters and Setters: Utilize getters and setters to control access to object properties and add validation.
- Consistent Naming Conventions: Follow naming conventions consistently:
- camelCase for variables and function names.
- PascalCase for class names.
- UPPER_SNAKE_CASE for constants.
Example: Complex Object with Best Practices
This example demonstrates how to create and manage complex objects using best practices, including classes, methods, and consistent naming conventions.
// Use PascalCase for class names
class Company {
constructor(name, location) {
this.name = name;
this.location = location;
this.employees = [];
}
// Method to add an employee
addEmployee(employee) {
this.employees.push(employee);
}
// Method to get names of all employees
getEmployeeNames() {
return this.employees.map(emp => emp.name);
}
}
// Use PascalCase for class names
class Employee {
constructor(name, position) {
this.name = name;
this.position = position;
}
}
// Use const for object declarations
const company = new Company("Tech Solutions", "New York");
const emp1 = new Employee("Alice", "Developer");
const emp2 = new Employee("Bob", "Designer");
company.addEmployee(emp1);
company.addEmployee(emp2);
console.log(company.getEmployeeNames()); // Output: [ 'Alice', 'Bob' ]
Explanation:
- Classes:
Company
andEmployee
classes are defined using PascalCase. - Object Management:
Company
class manages a list ofEmployee
objects. - Naming Conventions: Variable names and method names follow camelCase, and class names follow PascalCase.
- Best Practices:
const
is used to declare objects, ensuring their references remain unchanged.